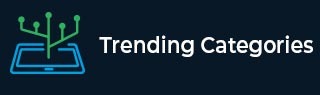
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to set logo inside loader using CSS?
To start with this question, we first need to create a ‘loader’. Any animation that informs a user or visitor that a page is now loading and will take a few seconds to finish loading is referred to as a loader.
The majority of the time, loaders come in handy when a website takes too long to retrieve the results. If a particular website doesn't have a CSS loader, the user will believe, it is not responding at all during the loading process.
Therefore, adding a CSS loader to a webpage causes the user to become distracted or wait a short while for the page to properly load. Rather than creating the impression that the site is unresponsive, a simple animation indicates that the website is still retrieving the results and that the web page will be ready in a few seconds.
You can make use of CSS to add style, animations or any other form of styling to create a loader.
Example
We will now use CSS to create the most common form of loader found across the internet.
<!DOCTYPE html> <html> <head> <title>Example of a loader using CSS</title> <style> h1 { color: rgb(0, 128, 111); } .loader { display: block; position: absolute; width: 15px; height: 15px; left: calc(50% - 1.25em); border-radius: 1.25em; transform-origin: 1.25em 2.25em; animation: rotateAnimation; animation-iteration-count: infinite; animation-duration: 1.85s; } @keyframes rotateAnimation { from { transform: rotateZ(0deg); } to { transform: rotateZ(360deg); } } .item1 { animation-delay: 0.12s; background-color: #1b7842; } .item2 { animation-delay: 0.22s; background-color: #239b53; } .item3 { animation-delay: 0.32s; background-color: #2ecc72; } .item4 { animation-delay: 0.42s; background-color: #82e0a0; } .item5 { animation-delay: 0.52s; background-color: #d5f5de; } </style> </head> <body> <center> <h1>Loader in CSS</h1> <h4>Example of the most common form of loader in CSS</h4> <div> <div class="loader item1"></div> <div class="loader item2"></div> <div class="loader item3"></div> <div class="loader item4"></div> <div class="loader item5"></div> </div> </center> </body> </html>
Example
Here is another form of loader that animates on the horizontal axis.
<!DOCTYPE html> <html> <head> <title>Example of a loader using CSS</title> <style> h1 { color: rgb(0, 128, 111); } .loader { display: block; position: absolute; width: 150px; height: 15px; left: calc(58% - 5.25em); transform-origin: 2px 20px; animation: rotateAnimation; animation-iteration-count: infinite; animation-duration: 2.85s; } @keyframes rotateAnimation { from { transform: rotateY(55deg); } to { transform: rotateY(360deg); } } .item1 { animation-delay: 0.12s; background-color: #1b7842; } .item2 { animation-delay: 0.22s; background-color: #239b53; } .item3 { animation-delay: 0.32s; background-color: #2ecc72; } .item4 { animation-delay: 0.42s; background-color: #82e0a0; } .item5 { animation-delay: 0.52s; background-color: #d5f5de; } </style> </head> <body> <center> <h1>Loader in CSS</h1> <h4>Example of the most common form of loader in CSS</h4> <div> <div class="loader item1"></div> <div class="loader item2"></div> <div class="loader item3"></div> <div class="loader item4"></div> <div class="loader item5"></div> </div> </center> </body> </html>
Adding the logo inside the loader
Now that we know how we can make a loader in CSS, we will now move on to how we can add a logo inside the loader we just created.
Let us first try to understand why we might need to add logo inside the loader. The logo is the mark and identity of a brand, and a brand adds a personal touch to the user experience. Now-a-days, all sites use a personalized loader which creates a positive impact for their brand whenever a user lands on their site.
Algorithm
The algorithm that we will follow to create the loader with logo embedded in it is given below −
Step 1 − In order to include our loader, we will build an HTML file and add an HTML div to the body of that file.
Step 2 − To give our logo and the loader animation effects, we will either write a CSS file or embed it using the style tag.
Step 3 − Insert the logo using the <img> tag within the div tag so that it now displays inside the loader class
The properties we could use for our loader are given below −
We will customize the border, color, height, width as per the logo that greatly improves the overall consistency and adds originality to the brand.
To add gradually changing animations we will make use of the @keyframes rules in CSS.
Then to make the loader rotate we will be using the transform property of CSS.
We will be styling the logo image to be of dimensions either smaller or equal to that of the loader. We will add the animation style in opposite direction so as to cancel out the effect of logo being rotated.
Example
Below is an example that uses the above algorithm to add loader to the website.
<!DOCTYPE html> <html lang="en"> <head> <style type="text/css"> .loaderClass { border: 12px solid #dcd7d7; border-top: 12px solid #04802f; border-radius: 50%; width: 120px; height: 120px; animation: SpinLoader 2.5s linear infinite; } .loaderClass img { height: 120px; width: 120px; border-radius: 50%; animation: LogoModify 2.5s linear infinite; } @keyframes SpinLoader { 0% { transform: rotate(0deg); } 100% { transform: rotate(360deg); } } @keyframes LogoModify { 0% { transform: rotate(360deg); } 100% { transform: rotate(0deg); } } </style> </head> <body> <div class="loaderClass"> <img src="https://www.tutorialspoint.com/static/images/client/science.svg" alt="logoImage"> </div> </body> </html>
Conclusion
To conclude, setting a logo inside your loader using CSS is an easy task. All you need to do is set the width and height of your logo, then use the "background-image" property to add it as background image of the loader. You can also adjust its position by using “background-position” or “margin” properties and customize it further with other styling options like border, box shadow etc. With some basic knowledge of CSS, you can easily create beautiful loaders with logos in no time!