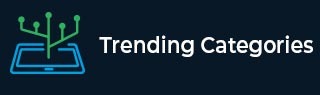
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to set a Variable to equal nothing in SASS?
SASS or Syntactically Awesome Style Sheets, is a very popular preprocessor scripting language among developers that are used to enhance the functionality of CSS. SASS allows the developers to use variables, nesting, mixins, and some other advanced features that are not available in CSS.
One of the key features of using SASS is the feature of declaring variables, which are nothing but placeholders for the values that we can reuse throughout our web application stylesheet. Variables help save time and effort for the developers enabling them to update multiple values at once just by making the code more readable and easier to maintain.
Sometimes, we may want to set a variable equal to nothing which in other languages means setting the variable as null or undefined. This is very useful when we want to create a placeholder variable that we may assign a value to later in the application. In this article, we’ll see how to set a Variable to equal nothing in SASS.
Pre-requisite
To run SASS in HTML, make sure you have a SASS compiler installed in your IDE like in VS Code, download and install the SASS compiler using the following command −
npm install -g sass
This will install the SASS compiler globally on your system. Next, create a new file with the extension .scss which will contain our SASS code.
After which, import the SASS file into the HTML file. In the HTML file, add a link to your SASS file using the ‘link’ tag in the head section of your HTML file. The link should have the rel attribute set to "stylesheet" and the href attribute set to the path of your SASS file.
Different ways to set a variable to equal nothing in SASS
To set the variable to equal nothing, there are multiple ways like using the null keyword, unset function, or #{} interpolation method. Let’s discuss all of these methods in detail one by one.
Method 1: Using the Null Keyword
The null keyword is used in SASS to represent the absence of a value. When a variable is set to null, it means that the variable has no value assigned to it. This can be useful in situations where you want to declare a variable but don't want to assign a value to it yet.
Syntax
To set a variable to equal nothing in SASS, simply use the null keyword like this −
$newVar: null;
Or, we can set a variable to null by assigning it to a variable that has been set to null
$newOtherVar: $newVar;
Example
Let’s see a complete example to see how to set a variable equal to nothing using the null keyword.
HTML Code
<!DOCTYPE html> <html> <head> <title>Example to set the variable to equal nothing using null keyword</title> <style> /* Add the CSS compiled code below */ </style> </head> <body> <h1>Welcome to Tutorialspoint!</h1> <p>The one stop solution to learn technology articles.</p> </body> </html>
SASS Code
Below is the SASS code, where we have declared the variable $newVar having the value equal to null.
$newVar: null; body { background-color: $newVar; color: $newVar; font-size: $newVar; }
Compiled CSS Code
Below is the compiled code that has been generated after compiling the above SASS code with the help of the SASS compilers. To see the changes in your document, make sure you add the below code between the <style> element in the respective HTML file.
body { background-color: ; color: ; font-size: ; }
Method 2: Using the Unset Ffunction
Another way to set a variable to equal nothing in SASS is to use the unset function. The unset function removes a variable from memory, effectively setting it to null. Here's how you can use the unset function to set a variable to null.
Syntax
$newVar: 15; $newVar: unset($newVar);
In the above syntax, $newVar is first assigned the value of 15. The unset function is then used to remove $newVar from memory, effectively setting it to null.
Example
Let’s see a complete example to see how to set a variable equal to nothing using the unset function.
HTML Code
<!DOCTYPE html> <html> <head> <title>Example to set the variable to equal nothing using unset function</title> <style> /* Add the CSS compiled code below */ </style> </head> <body> <h1>Welcome to Tutorialspoint!</h1> <p>The one stop solution to learn technology articles.</p> </body> </html>
SASS Code
Below is the SASS code, where we have declared two variables $newVar1 and $newVar2 having a value equal to 15 and 12 respectively. Now the unset function is used to remove $newVar1 and $newVar2 from memory, effectively setting them to null.
$newVar1: 15; $newVar1: 12; $newVar1: unset($newVar1); $newVar2: unset($newVar2); h1 { font-size: $newVar1; } p { font-size: $newVar2; }
Compiled CSS Code
Below is the compiled code that has been generated after compiling the above SASS code with the help of the SASS compilers. To see the changes in your document, make sure you add the below code between the <style> element in the respective HTML file.
h1 { font-size: ; } p { font-size: ; }
Method 3: Using #{} Interpolation
The #{} interpolation syntax is evaluated at compile time, so the resulting value of $newVar will be an empty string in the compiled CSS. This may not be the desired behavior in all cases, so it's important to use this technique carefully and only when it's appropriate.
Syntax
$newVar: #{" "};
In the above syntax, $newVar is set to an empty string, which is created using the #{} interpolation syntax with a single space inside. This technique can be useful in situations where you need to set a variable to an empty string, rather than null.
Example
Let’s see the example to set a variable equal to nothing using interpolation method.
HTML Code
<!DOCTYPE html> <html> <head> <title>Example to set the variable to equal nothing using Interpolation</title> <style> /* Add the CSS compiled code below */ </style> </head> <body> <h1>Welcome to Tutorialspoint!</h1> </body> </html>
SASS Code
Below is the SASS code, where we have declared a variable $newVar which is set to an empty string using the interpolation syntax.
$newVar: #{" "}; body { padding: $newVar; margin: $newVar; }
Compiled CSS Code
Below is the compiled code that has been generated after compiling the above SASS code with the help of the SASS compilers. To see the changes in your document, make sure you add the below code between the <style> element in the respective HTML file.
body { padding: ; margin: ; }
Conclusion
In this article, we have seen how to set a variable to equal nothing in SASS using different methods that include using the null keyword, the unset function, and the #{} interpolation syntax. Telling more about these techniques, they can be very useful in different situations or needs of the project where we need to create a placeholder variable that may be assigned a value later in the application.
In SASS, declaring the variables is more readable, and maintainable which saves the time and effort of the developers. To work with SASS, make sure to have a SASS compiler installed in your IDE like the process of using SASS in VS Code has also been discussed above where we need to import the SASS file into the HTML file correctly.