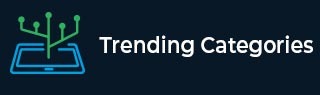
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to see if a widget exists in Tkinter?
To make a particular Tkinter application fully functional and operational, we can use as many widgets as we want. If we want to check if a widget exists or not, then we can use the winfo_exists() method. The method can be invoked with the particular widget we want to check. It returns a Boolean value where True(1) specifies that the widget exists in the application, and False(0) specifies that the widget doesn't exist in the application.
Example
# Import the required libraries from tkinter import * from tkinter import ttk # Create an instance of Tkinter Frame win = Tk() # Set the geometry win.geometry("700x250") # Define a function to check if a widget exists or not def check_widget(): exists = label.winfo_exists() if exists == 1: print("The widget exists.") else: print("The widget does not exist.") # Create a Label widget label = Label(win, text="Hey There! Howdy?", font=('Helvetica 18 bold')) label.place(relx=.5, rely=.3, anchor=CENTER) # We will define a button to check if a widget exists or not button = ttk.Button(win, text="Check", command=check_widget) button.place(relx=.5, rely=.5, anchor=CENTER) win.mainloop()
Output
Running the above code will display a window with a button and a label widget. In the application, we can check if the label widget is present or not.
If you click the button "Check", it will print whether the label widget exists or not.
The widget exists.
Advertisements