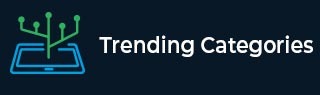
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to reverse a number in Python?
Reversing an integer number is an easy task. We may encounter certain scenarios where it will be required to reverse a number.
Input: 12345 Output: 54321
There are two ways, we can reverse a number −
Convert the number into a string, reverse the string and reconvert it into an integer
Reverse mathematically without converting into a string
Convert into string and Reverse
This method of reversing a number is easy and doesn’t require any logic. We will simply convert the number into string and reverse it and then reconvert the reversed string into integer. We can use any suitable method for reversing the string.
Example
def reverse(num): st=str(num) revst=st[::-1] ans=int(revst) return ans num=12345 print(reverse(num))
Output
54321
Reverse mathematically without converting into string
This method requires mathematical logic. This method can be used when there is a restriction of not converting the number into string.
Example
def reverse(num): rev=0 while(num>0): digit=num%10 rev=(rev*10)+digit num=num//10 return rev num=12345 print(reverse(num))
Output
54321
Advertisements