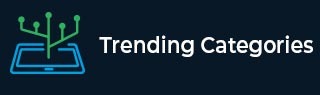
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to rethrow an exception in Java?
Sometimes we may need to rethrow an exception in Java. If a catch block cannot handle the particular exception it has caught, we can rethrow the exception. The rethrow expression causes the originally thrown object to be rethrown.
Because the exception has already been caught at the scope in which the rethrow expression occurs, it is rethrown out to the next enclosing try block. Therefore, it cannot be handled by catch blocks at the scope in which the rethrow expression occurred. Any catch blocks for the enclosing try block have an opportunity to catch the exception.
Syntax
catch(Exception e) { System.out.println("An exception was thrown"); throw e; }
Example
public class RethrowException { public static void test1() throws Exception { System.out.println("The Exception in test1() method"); throw new Exception("thrown from test1() method"); } public static void test2() throws Throwable { try { test1(); } catch(Exception e) { System.out.println("Inside test2() method"); throw e; } } public static void main(String[] args) throws Throwable { try { test2(); } catch(Exception e) { System.out.println("Caught in main"); } } }
Output
The Exception in test1() method Inside test2() method Caught in main
Advertisements