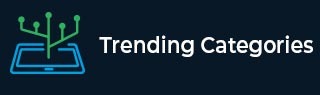
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to replace a specified element in slice of bytes in Golang?
In Golang, it is common to need to replace a specific element in a slice of bytes with a new value. Fortunately, there is a simple way to accomplish this using the built-in copy function. In this article, we'll explore how to replace a specified element in a slice of bytes in Golang.
Replacing a Specified Element in a Slice of Bytes in Golang
To replace a specified element in a slice of bytes in Golang, we first need to find the index of the element that we want to replace. Once we have the index, we can use the copy function to replace the element with a new value.
Example
Here's an example −
package main import "fmt" func main() { s := []byte{'a', 'b', 'c', 'd'} index := 2 newElement := byte('z') fmt.Printf("Original slice: %v\n", s) s[index] = newElement fmt.Printf("Slice after replacement: %v\n", s) }
In this example, we start with a slice of bytes s containing the values {'a', 'b', 'c', 'd'}. We want to replace the element at index 2 (the value c) with a new value of z.
To do this, we simply assign the new value to the element at the specified index. We then print the original slice and the updated slice to the console to show the difference.
Output
The output of this program will be −
Original slice: [97 98 99 100] Slice after replacement: [97 98 122 100]
As you can see, the value at index 2 of the original slice (the character c) has been replaced with the new value of z.
Conclusion
In this article, we've learned how to replace a specified element in a slice of bytes in Golang using the built-in copy function. By understanding this simple technique, you can easily replace elements in your own slices of bytes in Golang, allowing you to manipulate data in a flexible and efficient manner.