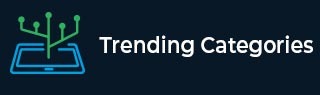
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to repeat a slice of bytes in Golang?
In Golang, repeating a slice of bytes is a common operation that can be useful in many different applications. Fortunately, the bytes package in Golang provides a simple way to repeat a slice of bytes multiple times. In this article, we'll explore how to repeat a slice of bytes in Golang.
Repeating a Slice of Bytes in Golang
To repeat a slice of bytes in Golang, we can use the bytes.Repeat function provided by the bytes package. The bytes.Repeat function takes two arguments: a slice of bytes to repeat, and the number of times to repeat the slice.
Example
Here's an example −
package main import ( "bytes" "fmt" ) func main() { b := []byte{'a', 'b', 'c'} repeated := bytes.Repeat(b, 3) fmt.Println(string(repeated)) }
In this example, we have a slice of bytes b containing the values {'a', 'b', 'c'}. We use the bytes.Repeat function to repeat the slice three times, and store the result in the repeated variable. Finally, we convert the repeated slice of bytes to a string and print it to the console.
Output
The output of this program will be −
abcabcabc
As you can see, the bytes.Repeat function repeats the slice of bytes three times, resulting in a new slice of bytes with the values {'a', 'b', 'c', 'a', 'b', 'c', 'a', 'b', 'c'}.
Conclusion
In this article, we've learned how to repeat a slice of bytes in Golang using the bytes.Repeat function provided by the bytes package. Repeating a slice of bytes can be useful in many different applications, and by understanding this simple technique, you can easily perform this operation in your own Golang projects.