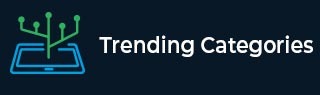
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to put a border around a Frame in Python Tkinter?
To put a border around a Frame in Tkinter, we have to use the highlightbackground and highlightthickeness parameters while creating the Frame. Let's take an example and see how to use these two parameters.
Steps −
Import the tkinter library and create an instance of tkinter frame.
Set the size of the frame using geometry() method.
Create a frame with Frame() method. Highlight the border of the frame with a color, highlightbackground="blue". Then, set the thickness of the border, highlightthickness=2.
Next, create some widgets inside the frame. In the example, we have placed four checkbuttons and a button inside the frame.
Finally, run the mainloop of the application window.
Example
from tkinter import * top = Tk() top.geometry("700x350") frame1 = Frame(top, highlightbackground="blue", highlightthickness=2) frame1.pack(padx=20, pady=20) C1 = Checkbutton(frame1, text = "Music", width=200, anchor="w") C1.pack(padx=10, pady=10) C2 = Checkbutton(frame1, text = "Video", width=200, anchor="w") C2.pack(padx=10, pady=10) C3 = Checkbutton(frame1, text = "Songs", width=200, anchor="w") C3.pack(padx=10, pady=10) C4 = Checkbutton(frame1, text = "Games", width=200, anchor="w") C4.pack(padx=10, pady=10) Button(frame1, text="Button-1", font=("Calibri",12,"bold")).pack(padx=10, pady=10) top.mainloop()
Output
It will produce the following output −
There's an easier method to create a simple border around a frame. Instead of having a Frame, create a LabelFrame and it will automatically set a border around the frame widgets.
Learn Python Programming: Python Tutorial