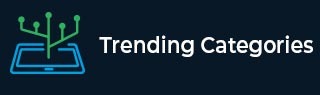
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to print the first character of each word in a String in Java?
A String class can be used to represent the character strings, all the string literals in a Java program are implemented as an instance of a String class. The Strings are constants and their values cannot be changed (immutable) once created.
We can print the first character of each word in a string by using the below program.
Example
public class FirstCharacterPrintTest { public static void main(String[] args) { String str = "Welcome To Tutorials Point"; char c[] = str.toCharArray(); System.out.println("The first character of each word: "); for (int i=0; i < c.length; i++) { // Logic to implement first character of each word in a string if(c[i] != ' ' && (i == 0 || c[i-1] == ' ')) { System.out.println(c[i]); } } } }
Output
The first character of each word: W T T P
Advertisements