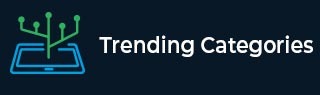
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to print out the contents of a vector in C++?
Vectors are similar to the dynamic arrays but vectors can resize. Vectors are sequence containers that can change their size according to the insertion or deletion of elements. Containers are the objects which holds the data of same type.
Vectors may allocate some extra storage for the future growth of elements in the vector. Vector elements are stored in the contiguous memory. The data is entered at the end of vector.
Here is an example to print the contents of a vector in C++ language,
Example
#include<iostream> #include<vector> void print(std::vector <int> const &a) { std::cout << "The vector elements are : "; for(int i=0; i < a.size(); i++) std::cout << a.at(i) << ' '; } int main() { std::vector<int> a = {2,4,3,5,6}; print(a); return 0; }
Output
Here is the output −
The vector elements are : 2 4 3 5 6
In the above program, function print() contains the code to get the elements of vector. In the for loop, size of vector is calculated for the maximum number of iterations of loop and using at(), the elements are printed.
for(int i=0; i < a.size(); i++) std::cout << a.at(i) << ' ';
In the main() function, the elements of vector are passed to print them.
std::vector<int> a = {2,4,3,5,6}; print(a);