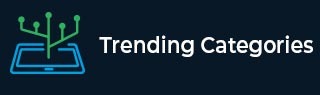
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to pause and play a loop using event listeners in JavaScript?
In this article, we are going to understand how we can pause and play a loop whenever we want using event listeners and promises in JavaScript. Pause and play to a loop refers to as a for loop is running and we want to make a pause in between any position in a loop or want to play it again.
An event listener can be attached to any element to control how the event reacts to bubbling. It attaches an event handler to an element.
Syntax
Following is the syntax of an event listener −
element.addEventListener(event, function, useCapture);
Parameters
event − it is the name of the event i.e. “click”.
function − the function to return when the event happens.
useCapture − Optional, default is false.
Approach
To pause and play a loop we will use event listeners with the concept of the promise.
To achieve this task, we are using a function named eventt, this function is using a promise which will resolve whenever the loop is paused or played.
It is getting paused using removeAttribute and setAttribute functions, there is a variable named as go which is used to signify whenever we play or pause the loop, go=1 signifies the loop is paused and go=0 signifies the loop is played.
In the last, we are using an async-await function which is used to start the for loop and display whenever the loop is paused or played.
Example
We are using the following code to play and pause a loop in JavaScript using event listeners.
<!DOCTYPE html> <html> <body> <h3>Pause and play a loop using event Listeners</h3> <p>Click on below buttons to pause and play the counter</p> <button id="start">Play</button> <button id="stop">Pause</button> <div> <p id="sec">0</p> </div> <script> document.getElementById("start") .setAttribute("disabled", "true") var go = 0; function waitforme(ms) { return new Promise(resolve => { setTimeout(() => { resolve('') }, ms); }) } function eventt() { return new Promise(resolve => { let playbuttonclick = function () { document.getElementById("stop") .removeAttribute("disabled") document.getElementById("start") .setAttribute("disabled", "true") document.getElementById("start") .removeEventListener("click", playbuttonclick); go = 0; resolve("resolved"); } document.getElementById("start") .addEventListener("click", playbuttonclick) }) } document.getElementById("stop") .addEventListener("click", function () { go = 1; document.getElementById("stop") .setAttribute("disabled", "true") document.getElementById("start") .removeAttribute("disabled") }) async function calculate() { for (let a = 0;a<10000;a++) { document.getElementById("sec").innerHTML = a ; await waitforme(1000); if (go == 1) await eventt(); } } calculate(); </script> </body> </html>
Here you can see the output the loop will start from index zero and will stop whenever you press the pause button and start again whenever you press the play button. Here the numbers are running to the same speed as seconds move but we can also increase the speed of the counting by changing values in the await waitforme() function. We can also display more than one counting in a single loop by adding some functions to display the minutes.
Note − The value in the await wait for me function has the unit as milliseconds means value 1000=1sec.