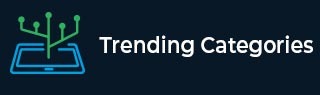
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How To Password Protect A Page Using Only HTML, CSS And JavaScript?
Password protection is an important security measure for webpages that contain sensitive information or require authentication for access. If you want to add extra security to a web page without using a server-side language, you can use HTML, CSS, and JavaScript to password protect the page.
This article will show you how to create a simple form that requires users to enter a password before they can view the content of your protected page. Let’s look into the following examples for getting better understanding on protecting a page with password.
Example
In the following example, we are running the script and protecting the webpage; if the user tries to access the webpage, it will prompt them to enter a password.
<!DOCTYPE html> <html> <body> <script> var password = "tutorial"; (function passcodeprotect() { var passcode = prompt("Enter PassCode"); while (passcode !== password) { alert("Incorrect PassCode"); return passcodeprotect(); } }()); alert('Welcome To The TP..!'); </script> </body> </html>
When the script gets executed, it will generate an output displaying an alert asking to enter the password. When the user matches the password (“tutorial”), it will display a message; otherwise, an incorrect password will be displayed on the webpage.
Example
Considering the following example, where we are creating an input field for a typed password to protect the webpage along with a click button.
<!DOCTYPE HTML> <html> <body> <center> <input type="password" placeholder="passcode" id="tutorial"> <button onclick="protectpasscode()">CHECK</button> <script> function protectpasscode() { const result = document.getElementById("tutorial").value; let passcode = 12345; let space = ''; if (result == space) { alert("Type passcode") } else { if (result == passcode) { document.write("<center><h1>TP, The Best E-Learning </h1></center>"); } else { alert("Incorrect Passcode"); location.reload(); } } } </script> </center> </body> </html>
On running the above script, the output window will pop up, displaying the input field for typing a password along with a click button on the webpage. If the user matches the passcode (which is 12345), it will open the form consisting of text; otherwise, it will display an incorrect passcode.
Example
Execute the below example, where we are running the script to protect the webpage from displaying its content after executing the script.
<!DOCTYPE html> <html> <body style="background-color:#EAFAF1"> Enter Password: <input type='text' value='' id='input'><br><br> <input type='checkbox' onclick='protectpasscode()'>Show results <p id='tutorial' style='display:none; color: black;'>Mahendra Singh Dhoni, also known as MS Dhoni, is an Indian former international cricketer who was captain of the Indian national cricket team in limited-overs formats from 2007 to 2017 and in Test cricket from 2008 to 2014. </p> <script> function protectpasscode() { var a = document.getElementById('input'); var b = document.getElementById('tutorial'); if (a.value === '54') { b.style.display = 'block'; } else { b.style.display = 'none'; } } </script> </body> </html>
When the script gets executed, it will generate an output displaying an input field to enter a password and a toggle checkbox on the webpage. When the user matches the password, (which is 54) and toggles the checkbox, the content inside the webpage is displayed.