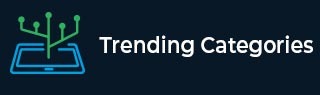
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to order the results of related models in Laravel Eloquent?
Laravel Eloquent comes with the orderBy() method that can be used to order the results from the model. We are going to make use of Users table as shown below −
Let us create another table user_roles with following data −
Example 1
Following examples show how to make use of orderBy to order results.
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Models\User; use Illuminate\Support\Str; //use Illuminate\Support\Facades\DB; class UserController extends Controller{ public function index() { $users = User::orderBy('name', 'ASC')->take(10)->get(); foreach ($users as $user) { echo $user->name."<br/>"; } } }
In above example the query used is as follows −
SELECT name from users order by name ASC limit 10;
The output in mysql is as follows −
Output
The output when you check in browser for Laravel is −
Example 2
In this example we are going to see how to use order by joining two tables. The tables being used are users and user_roles tables.
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Models\User; class UserController extends Controller{ public function index() { $users = User::join('user_roles', 'users.id', '=', 'user_roles.user_id') ->orderBy('users.name', 'ASC') ->select('users.*') ->paginate(10); foreach ($users as $user) { echo $user->name."<br/>"; } } }
Output
The output of the above code is as follows −
The query for the above example is −
SELECT u.* From users u INNER JOIN user_roles ur ON u.id = ur.user_id ORDER BY u.name ASC;
The output in mysql is −
Example 3
Another example of using orderBy to get records in descending order is as follows −
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Models\User; use Illuminate\Support\Str; //use Illuminate\Support\Facades\DB; class UserController extends Controller{ public function index() { $users = User::orderBy('id', 'desc')->select('users.*') ->take(10)->get(); foreach ($users as $user) { echo $user->name."<br/>"; } } }
Output
The output of the above code is −
Example 4
Using orderBy on name to get records in descending order
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Models\User; use Illuminate\Support\Str; //use Illuminate\Support\Facades\DB; class UserController extends Controller{ public function index() { $users = User::orderBy('name', 'desc')->select('users.*') ->take(10)->get(); foreach ($users as $user) { echo $user->name."<br/>"; } } }
Output
The output of the above code is −