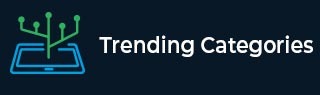
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to make use of Join with LINQ and Lambda in C#?
Inner join returns only those records or rows that match or exists in both the tables. We can also apply to join on multiple tables based on conditions as shown below. Make use of anonymous types if we need to apply to join on multiple conditions.
In the below example we have written 2 ways that can be used to join in Linq Here the Department and the Employee are joined
Example
class Program{ static void Main(string[] args){ var result = Employee.GetAllEmployees().Join(Department.GetAllDepartments(), e => e.DepartmentID, d => d.ID, (employee, department) => new{ EmployeeName = employee.Name, DepartmentName = department.Name }); foreach (var employee in result){ Console.WriteLine(employee.EmployeeName + "\t" + employee.DepartmentName); } var result1 = from e in Employee.GetAllEmployees() join d in Department.GetAllDepartments() on e.DepartmentID equals d.ID select new{ EmployeeName = e.Name, DepartmentName = d.Name }; foreach (var employee in result1){ Console.WriteLine(employee.EmployeeName + "\t" + employee.DepartmentName); } Console.ReadLine(); } } public class Employee{ public int ID { get; set; } public string Name { get; set; } public int DepartmentID { get; set; } public static List<Employee> GetAllEmployees(){ return new List<Employee>(){ new Employee { ID = 1, Name = "A", DepartmentID = 1 }, new Employee { ID = 2, Name = "B", DepartmentID = 2 }, new Employee { ID = 3, Name = "B", DepartmentID = 1 }, new Employee { ID = 4, Name = "V", DepartmentID = 1 }, new Employee { ID = 5, Name = "F", DepartmentID = 2 }, new Employee { ID = 6, Name = "R", DepartmentID = 2 }, new Employee { ID = 7, Name = "TT", DepartmentID = 1 }, new Employee { ID = 8, Name = "YY", DepartmentID = 1 }, new Employee { ID = 9, Name = "WW", DepartmentID = 2 }, new Employee { ID = 10, Name = "QQ"} }; } } public class Department{ public int ID { get; set; } public string Name { get; set; } public static List<Department> GetAllDepartments(){ return new List<Department>(){ new Department { ID = 1, Name = "IT"}, new Department { ID = 2, Name = "HR"}, new Department { ID = 3, Name = "Contract"}, }; } }
Output
A IT B HR B IT V IT F HR R HR TT IT YY IT WW HR A IT B HR B IT V IT F HR R HR TT IT YY IT WW HR
Advertisements