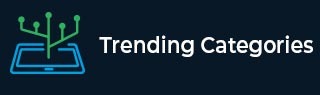
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to make elements of array immutable in Java?
No, you cannot make the elements of an array immutable.
But the unmodifiableList() method of the java.util.Collections class accepts an object of the List interface (object of implementing its class) and returns an unmodifiable form of the given object. The user has only read-only access to the obtained list.
And the asList() method of the ArrayList class accepts an array and returns a List object.
Therefore, to convert an array immutable −
Obtain the desired array.
Convert it into a list object using the asList() method.
Pass the obtained list as a parameter to the unmodifiableList() method.
Example
import java.util.Arrays; import java.util.Collections; import java.util.List; public class UnmodifiableExample { public static void main(String args[]) { //Creating a string array String strArray[] = {"Raju", "Rama", "Rahman", "Rachel", "Ranbhir", "Rangan"}; //Converting the string array to list object List<String> list = Arrays.asList(strArray); //Converting the List object to immutable List<String> immutable = Collections.unmodifiableList(list); System.out.println(immutable); immutable.add("komala"); } }
Output
[Raju, Rama, Rahman, Rachel, Ranbhir, Rangan] Exception in thread "main" java.lang.UnsupportedOperationException at java.util.Collections$UnmodifiableCollection.add(Unknown Source) at September19.UnmodifiableExample.main(UnmodifiableExample.java:19)
Advertisements