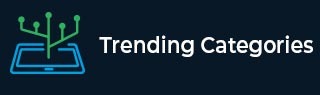
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to list out the hidden files in a Directory using Java program?
The class named File of the java.io package represents a file or directory (path names) in the system. This class provides various methods to perform various operations on files/directories.
The isHidden() method of the File class verifies weather the (abstract path of) file/directory represented by the current File object, is hidden.
The ListFiles() method of the File class returns an array holding the objects (abstract paths) of all the files (and directories) in the path represented by the current (File) object.
Therefore, to list all the hidden files in a directory get all the file objects using the ListFiles() method, verify weather each file is hidden using the isHidden() method.
Example
Following Java program prints the file name and path of all the hidden files and directories in the specified directory −
import java.io.File; public class ListingHiddenDirectories { public static void main(String args[]) { String filePath = "D://ExampleDirectory//"; //Creating the File object File directory = new File(filePath); //List of all files and directories File filesList[] = directory.listFiles(); System.out.println("List of files and directories in the specified directory:"); for(File file : filesList) { if(file.isHidden()) { System.out.println("File name: "+file.getName()); System.out.println("File path: "+file.getAbsolutePath()); } } } }
Output
List of files and directories in the specified directory: File name: hidden_directory1 File path: D:\ExampleDirectory\hidden_directory1 File name: hidden_directory2 File path: D:\ExampleDirectory\hidden_directory2 File name: SampleHiddenfile1.txt File path: D:\ExampleDirectory\SampleHiddenfile1.txt File name: SampleHiddenfile2.txt File path: D:\ExampleDirectory\SampleHiddenfile2.txt File name: SampleHiddenfile3.txt File path: D:\ExampleDirectory\SampleHiddenfile3.txt