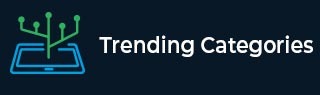
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to inject a service into the component in Angular 8?
In Angular, services are singleton objects which normally get instantiated only once for the entire angular application. Every service contains several methods and that includes some functionality to do. It is a mechanism to share functionality or responsibility within one or more than components.
We know, using Angular we can create nested-based component. Once our applications are nested, we may need to use the same functionality. Instead of writing same code in multiple components. We can write one place and we can share data with different components. The best way to use service to handle this.
In first versions of angular, we used to create services using providers, factories, delegates, values, etc. and also it is clear to which one need to use. But, in angular 8 it makes it clear to create services by two following ways.
Creating service with @Injector decorator
Register the class with provider or inject the class by using dependency injection.
Let’s look below, how to define a service in our application.
We can create our own services based on the requirement. To create a service, follow this steps.
First create one service.ts file with the proper name.
In this file, import Injectable from @angular/core package and provide @Injectable decorators at the beginning of the class.
Export the class object. So that we can this service in other components.
Write business logic based on the requirement inside export the class object.
Note
When we have our own service with provider metadata, we need to import in app.module.ts file to utilize those service functionalities. If we didn’t import created service it is not visible to the whole application. If we import created service only one component without importing it into main module, then that service only available to that particular component. If we provide the service in main module, for entire application only instance will be created for the service.
Example
Create a service class inside the app folder as messageService.service.ts.
Write inside the created service class like given below.
import { Injectable } from '@angular/core'; @Injectable() export class MessageService { constructor() { } sendMessage(message: string) { return "Hello"; } };
Here, @Injectable decorator converts a plain Typescript class into Angular service.
Register Service
To use dependency injection, service needs to be registered. Angular provides multiple option to register a service.
ModuleInjector @ root level.
ModuleInjector @ platform level.
ElementInjector using providers meta data.
ElementInjector using viewProviders meta data.
NullInjector.
Dependency Injection
Dependency Injection is one of the main benefits of angular framework. With the help of this DI, we can inject any types of dependency like service, external module in our application. For doing this, we do not even want to know how those dependency modules or services have been developed.
In angular, Dependency Injection contains three sections like Injector, Provider & Dependency.
Injector is used to reveal an object or APIs which basically helps us to create instances of the dependent class or services.
Provider is used to provide instruction to the injector how to create instances of dependent objects. The provider always taken the token value as input and then map that token value with the newly created instances of the class objects. Basically, it works like an instructor.
Dependency is used to identify the nature of the created objects.
Using DI,
We can create instances of the service classes in the constructor level using the provider metadata.
DI provides reference code of instance of the dependent class when we require it.
All the instances of the dependency injected objects are created as a Singleton object.
Let’s see examples of normal service and injecting services using providers.
Example: Inject into module
Create a service the class and add some functionality.
Here I am using the same service created in app folder i.e. messageService.ts file.
import { Injectable } from '@angular/core'; @Injectable(){ providedIn: "root" } export class MessageService { constructor() { } sendMessage (message: string) { return "Hello! Message from Angular Service"; } };
We have service class now import into app.module.ts file.
import { BrowserModule } from "@angular/platform-browser"; import { NgModule } from "@angular/core"; import { AppComponent } from "./app.component"; import { MessageService } from "./messageService"; @NgModule({ declarations: [AppComponent], imports: [BrowserModule], providers: [MessageService], bootstrap: [AppComponent] }) export class AppModule {}
Now, let’s import service class and get data from the service and display in UI.
Write the code in app.component.ts file as given below.
import { Component } from '@angular/core'; import { MessageService } from './messageService'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { message: string = ""; constructor(private messageService: MessageService ) {} ngOnInit() { this.message = this.messageService.sendMessage(); } }
In app.component.html file
<p>Service Example</p> <p>{{message}}</p>
Output
Service Example Hello! Message from Angular Service
Example: Inject service into component
Service class: messageService.ts file
import { Injectable } from "@angular/core"; @Injectable() export class MessageService { constructor() {} sendMessage(message: string) { return "Message from Angular Service"; } }
Here, root is not specifying because we want to use this service in particular component. Not as root service. So, no need to import in app.module.ts file.
Directly inject into the required component. Here I am injecting service class into app.component.ts file.
import { Component } from '@angular/core'; import { MessageService } from './messageService'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { message: string = ""; constructor(private messageService: MessageService ) {} ngOnInit() { this.message = this.messageService.sendMessage(); } }
In app.component.html file. Write code as given below.
<p>Service Example</p> <p>{{message}}</p>
Here, we can see, we injected service classes into module level or into only component. If we inject service into any particular component, we will be able to utilize only that particular component only.
Output
Service Example Message from Angular Service
Above, we discussed services, Dependency injection, how to inject services. Services are used to create shared data. We can achieve code reusability also by injecting dependencies services into components, and directives.