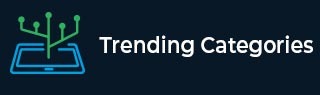
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to incorporate TestNG assertions in validating Response in Rest Assured?
We can incorporate TestNG assertions in validating Response in Rest Assured. To work with TestNG we have to add the below dependency in the pom.xml in our Maven project. The link to this dependency is available in the below link −
https://mvnrepository.com/artifact/org.testng/testng
To verify a response with a TestNG assertion, we need to use the methods of the Assert class. We shall first send a GET request via Postman on a mock API URL and go through the response.
Example
Using Rest Assured and TestNG, we shall verify the value of the Course field which is Automation Testing.
Code Implementation
import org.testng.Assert; import org.testng.annotations.Test; import static io.restassured.RestAssured.*; import io.restassured.RestAssured; import io.restassured.path.json.JsonPath; import io.restassured.response.Response; import io.restassured.response.ResponseBody; import io.restassured.specification.RequestSpecification; public class NewTest { @Test void verifyTestNg() { String c = "Automation Testing"; //base URI with Rest Assured class RestAssured.baseURI = "https://run.mocky.io/v3"; //input details RequestSpecification h = RestAssured.given(); //get response Response r = h.get("/e3f5da9c-6692-48c5-8dfe-9c3348cfd5c7"); //Response body ResponseBody bdy = r.getBody(); //convert response body to string String b = bdy.asString(); //JSON Representation from Response Body JsonPath j = r.jsonPath(); //Get value of Location Key String l = j.get("Course"); System.out.println("Course name: " + l); Assert.assertEquals(l, c); } }
Output
Advertisements