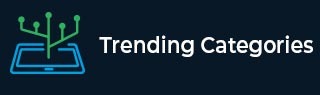
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to Implement Validation for RESTful Services with Spring
As more and more applications move towards a RESTful architecture, it becomes increasingly important to ensure that the data being exchanged between the client and the server is valid. In this article, we will look at how to implement validation for RESTful services with Spring.
What is Validation?
Validation is the process of checking that the data being inputted or returned is valid and conforms to a set of predefined rules. These rules can be simple, such as checking that a field is not empty, or more complex, such as ensuring that a credit card number is valid.
Why is Validation Important?
Validation is important for several reasons. Firstly, it ensures that the data being exchanged between the client and server is accurate and correct. This is important for maintaining data integrity and ensuring that the application works as expected.
Secondly, validation helps to prevent security vulnerabilities. By validating input data, we can prevent attacks such as SQL injection, cross-site scripting (XSS), and other attacks that can be caused by malformed input.
Thirdly, validation helps to provide a better user experience. By ensuring that the data being inputted is valid, we can provide better error messages and feedback to the user, allowing them to correct any mistakes and continue with the application flow.
Implementing Validation with Spring
Spring provides several tools for implementing validation in a RESTful service. In this section, we will look at some of these tools and how they can be used.
Validation Annotations
One of the easiest ways to implement validation in Spring is by using validation annotations. These annotations can be added to the fields of a request object and will automatically trigger validation when the request is received.
For example, let's say we have a RESTful service that accepts a user object with a name, email, and password. We can use the @NotBlank and @Email annotations to ensure that these fields are not empty and that the email is in a valid format.
public class User { @NotBlank private String name; @NotBlank @Email private String email; @NotBlank private String password; // Getters and setters }
When the request is received, Spring will automatically validate the user object and return an error response if any of the fields fail validation.
Custom Validators
While validation annotations are powerful, sometimes we need more complex validation rules that cannot be achieved with annotations alone. In these cases, we can create custom validators that implement our own validation logic.
To create a custom validator, we need to implement the Validator interface provided by Spring. This interface has two methods: supports() and validate().
The supports() method checks whether the validator supports the given class, while the validate() method performs the actual validation.
For example, let's say we have a RESTful service that accepts a product object with a name and price. We want to ensure that the name is not empty and that the price is greater than zero. We can create a custom validator to implement this validation logic.
public class ProductValidator implements Validator { @Override public boolean supports(Class<?> clazz) { return Product.class.equals(clazz); } @Override public void validate(Object target, Errors errors) { Product product = (Product) target; if (StringUtils.isBlank(product.getName())) { errors.rejectValue("name", "name.empty"); } if (product.getPrice() <= 0) { errors.rejectValue("price", "price.invalid"); } } }
In this example, we check that the name field is not empty using the StringUtils.isBlank() method, and that the price field is greater than zero. If any of these checks fail, we add an error to the Errors object using the rejectValue() method.
To use this custom validator, we need to register it with Spring. We can do this by adding it to the ValidatorFactoryBean.
@Configuration public class ValidationConfig { @Bean public ValidatorFactoryBean validatorFactoryBean() { ValidatorFactoryBean validatorFactoryBean = new ValidatorFactoryBean(); validatorFactoryBean.setValidationMessageSource(messageSource()); return validatorFactoryBean; } @Bean public MessageSource messageSource() { ResourceBundleMessageSource messageSource = new ResourceBundleMessageSource(); messageSource.setBasename("validation-messages"); return messageSource; } }
In this example, we create a ValidationConfig class and define a ValidatorFactoryBean bean. We also create a MessageSource bean that contains our validation error messages.
To use this custom validator in our RESTful service, we need to add the @Validated annotation to our controller method and the @Valid annotation to our request object.
@RestController @RequestMapping("/products") @Validated public class ProductController { @PostMapping public ResponseEntity<Product> createProduct(@Valid @RequestBody Product product) { // Create product and return response } }
In this example, we use the @Validated annotation on our controller class to enable method-level validation. We also use the @Valid annotation on our request object to trigger validation when the request is received.
Handling Validation Errors
When validation errors occur, we need to provide meaningful error messages to the user. Spring provides several ways to handle validation errors, including custom error messages and global error handling.
Custom Error Messages
We can provide custom error messages for each validation error by creating a validation-messages.properties file in our resources folder. This file should contain a key-value pair for each validation error message.
For example, let's say we have a validation error message for an empty product name field. We can add the following key-value pair to our validation-messages.properties file.
name.empty=Product name cannot be empty
When the validation error occurs, Spring will look up the error message using the key and return the corresponding message to the user.
Global Error Handling
Sometimes, we may want to handle all validation errors in a central location, rather than returning them to the user directly. We can do this by using Spring's GlobalExceptionHandler.
@ControllerAdvice public class GlobalExceptionHandler { @ExceptionHandler(MethodArgumentNotValidException.class) @ResponseStatus(HttpStatus.BAD_REQUEST) @ResponseBody public ErrorResponse handleValidationException(MethodArgumentNotValidException ex) { List<String> errors = ex.getBindingResult().getFieldErrors().stream() .map(error -> error.getField() + " " + error.getDefaultMessage()) .collect(Collectors.toList()); return new ErrorResponse("Validation error", errors); } }
In this example, we create a GlobalExceptionHandler class that handles MethodArgumentNotValidException exceptions. This exception is thrown when a validation error occurs.
We return a custom ErrorResponse object that contains the error message and a list of error strings. We use the getBindingResult() method to retrieve the errors from the exception and format them into a list.
Grouping Constraints
Sometimes we may want to group constraints together to apply them to a specific subset of fields. For example, we may want to apply a set of constraints to the user's personal information fields and a different set of constraints to their payment information fields.
We can do this by creating groups of constraints and annotating the fields with the appropriate group annotation.
public class User { @NotBlank(groups = PersonalInfo.class) private String firstName; @NotBlank(groups = PersonalInfo.class) private String lastName; @NotBlank(groups = PaymentInfo.class) private String creditCardNumber; @NotBlank(groups = PaymentInfo.class) private String cvv; // Getters and setters }
In this example, we create two interface groups: PersonalInfo and PaymentInfo. We annotate the fields with the appropriate group annotations and use the @Validated annotation to specify the group we want to validate.
Conclusion
Validation is an essential part of any RESTful service. By implementing validation with Spring, we can ensure that the data being exchanged between the client and server is valid, secure, and provides a good user experience.
Spring provides several tools for implementing validation, including validation annotations and custom validators. We can handle validation errors using custom error messages or global error handling.
By following these best practices, we can create robust and reliable RESTful services that provide value to our users and stakeholders.