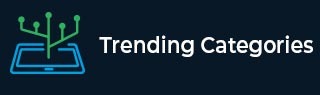
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to implement ToDoubleBiFunction using lambda expression in Java?
ToDoubleBiFunction<T, U> is a functional interface defined in java.util.function package. This functional interface accepts two parameters as input and produces a double-valued result. ToDoubleBiFunction<T, U> interface can be used as an assignment target for a lambda expression or method reference. This interface contains only one abstract method: applyAsDouble() and doesn't contain any default or static methods.
Syntax
@FunctionalInterface interface ToDoubleBiFunction<T, U> { double applyAsDouble(T t, U u); }
Example
import java.util.function.ToDoubleBiFunction; public class ToDoubleBiFunctionTest { public static void main(String args[]) { ToDoubleBiFunction<Integer, Integer> test = (t, u) -> t / u; // lambda expression System.out.println("The division of t and u is: " + test.applyAsDouble(50, 5)); System.out.println("The division of t and u is: " + test.applyAsDouble(100, 3)); } }
Output
The division of t and u is: 10.0 The division of t and u is: 33.0
Advertisements