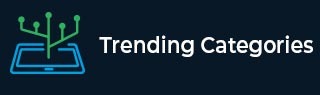
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to implement the encapsulation concept in JShell in Java 9?
Java Shell (simply JShell) is a REPL interactive tool for learning the Java and prototyping Java code. It evaluates declarations, statements, and expressions as entered and immediately prints out the result and runs from the command-line.
Encapsulation is an important concept in Java to make sure that "sensitive" data has been hidden from users. To achieve this, we must declare a class variable as private and provides public access to get and set methods and update the value of a private variable.
In the below code snippet, we have implemented the Encapsulation concept for Employee class.
jshell> class Employee { ...> private String firstName; ...> private String lastName; ...> private String designation; ...> private String location; ...> public Employee(String firstName, String lastName, String designation, String location) { ...> this.firstName = firstName; ...> this.lastName = lastName; ...> this.designation = designation; ...> this.location = location; ...> } ...> public String getFirstName() { ...> return firstName; ...> } ...> public String getLastName() { ...> return lastName; ...> } ...> public String getJobDesignation() { ...> return designation; ...> } ...> public String getLocation() { ...> return location; ...> } ...> public String toString() { ...> return "Name = " + firstName + ", " + lastName + " | " + ...> "Job designation = " + designation + " | " + ...> "location = " + location + "."; ...> } ...> } | created class Employee
In the below code snippet, we have created an instance of Employee class, and it prints out a name, designation, and location.
jshell> Employee emp = new Employee("Jai", "Adithya", "Content Developer", "Hyderabad"); emp ==> Name = Jai, Adithya | Job designation = Content Developer | location = Hyderabad.
Advertisements