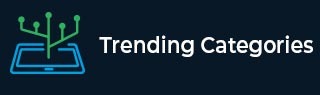
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to implement Single Responsibility Principle using C#?
A class should have only one reason to change.
Definition − In this context, responsibility is considered to be one reason to change.
This principle states that if we have 2 reasons to change for a class, we have to split the functionality in two classes. Each class will handle only one responsibility and if in the future we need to make one change we are going to make it in the class which handles it. When we need to make a change in a class having more responsibilities the change might affect the other functions related to the other responsibility of the class.
Example
Code Before the Single Responsibility Principle
using System; using System.Net.Mail; namespace SolidPrinciples.Single.Responsibility.Principle.Before { class Program{ public static void SendInvite(string email,string firstName,string lastname){ if(String.IsNullOrWhiteSpace(firstName)|| String.IsNullOrWhiteSpace(lastname)){ throw new Exception("Name is not valid"); } if (!email.Contains("@") || !email.Contains(".")){ throw new Exception("Email is not Valid!"); } SmtpClient client = new SmtpClient(); client.Send(new MailMessage("Test@gmail.com", email) { Subject="Please Join the Party!"}) } } }
Code After Single Responsibility Principle
using System; using System.Net.Mail; namespace SolidPrinciples.Single.Responsibility.Principle.After{ internal class Program{ public static void SendInvite(string email, string firstName, string lastname){ UserNameService.Validate(firstName, lastname); EmailService.validate(email); SmtpClient client = new SmtpClient(); client.Send(new MailMessage("Test@gmail.com", email) { Subject = "Please Join the Party!" }); } } public static class UserNameService{ public static void Validate(string firstname, string lastName){ if (string.IsNullOrWhiteSpace(firstname) || string.IsNullOrWhiteSpace(lastName)){ throw new Exception("Name is not valid"); } } } public static class EmailService{ public static void validate(string email){ if (!email.Contains("@") || !email.Contains(".")){ throw new Exception("Email is not Valid!"); } } } }
Advertisements