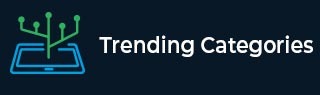
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to implement PropertyChangeListener using lambda expression in Java?
A PropertyChangeListener is a functional interface from java.beans package. It has one abstract method propertyChange() and gets called when a bound property is changed. This method takes a PropertyChangeEvent argument that has details about an event source and the property that has changed. A PropertyChangeSupport can be used by beans that support bound properties. It can manage a list of listeners and dispatches property change events. The PropertyChangeListener's implementer does the role of an Observer and bean wrapping the PropertyChangeSupport is Observable.
Syntax
void propertyChange(PropertyChangeEvent evt)
Example
import java.beans.*; import java.awt.*; import java.awt.event.*; import javax.swing.*; import javax.swing.border.*; public class PropertyChangeListenerLambdaTest { public static void main(String [] args) { new PropertyChangeListenerLambdaTest(); } public PropertyChangeListenerLambdaTest() { JFrame frame = new JFrame("First Frame"); final JLabel label = new JLabel("Observing..."); label.setFont(new Font("Dialog", Font.PLAIN, 18)); frame.add(label); frame.getRootPane().setBorder(new EmptyBorder(10, 10, 10, 10)); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setSize(250, 150); frame.setLocation(200, 200); frame.setVisible(true); MessageBean bean = new MessageBean(); bean.addPropertyChangeListener(e -> // lambda expression label.setText((String) e.getNewValue()) ); new Frame1(bean); } private class Frame1 { private int clicks; Frame1(MessageBean bean) { JFrame frame = new JFrame("Second Frame"); JLabel label = new JLabel("Click anywhere to fire a property change event"); label.setFont(new Font("Dialog", Font.PLAIN, 18)); frame.add(label); frame.getRootPane().setBorder(new EmptyBorder(10, 10, 10, 10)); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setSize(450, 150); frame.setLocation(600, 200); frame.setVisible(true); frame.addMouseListener(new MouseAdapter() { @Override public void mouseClicked(MouseEvent e) { String data = "Click-count [" + ++clicks + "]"; bean.setValue(data); } }); } } } class MessageBean { private final PropertyChangeSupport support = new PropertyChangeSupport(this); private String value; public void addPropertyChangeListener(PropertyChangeListener listener) { support.addPropertyChangeListener(listener); } public void removePropertyChangeListener(PropertyChangeListener listener) { support.removePropertyChangeListener(listener); } public String getValue() { return value; } public void setValue(String newValue) { String oldValue = value; value = newValue; support.firePropertyChange("value", oldValue, newValue); } }
Output
Advertisements