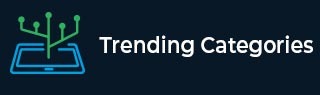
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to get the width of the Tkinter widget?
Tkinter widgets are supposed to be present in the Tkinter application window. All the widgets can be configured and customized by using predefined properties or functions.
To get the width of a widget in a Tkinter application, we can use winfo_width() method. It returns the width of the widget which can be printed later as the output.
Example
#Import the required libraries from tkinter import * #Create an instance of Tkinter Frame win = Tk() #Set the geometry win.geometry("700x350") #Set the default color of the window win.config(bg='#aad5df') #Create a Label to display the text label=Label(win, text= "Hello World!",font= ('Helvetica 18 bold'), background= 'white', foreground='purple1') label.pack(pady = 50) win.update() #Return and print the width of label widget width = label.winfo_width() print("The width of the label is:", width, "pixels") win.mainloop()
Output
Running the above code will display a window that contains a Label widget.
When we compile the code, it will print the width of the label widget on the console.
The width of the label is: 148 pixels
Advertisements