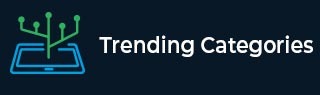
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to get the style of current selection in Text using FabricJS?
In this tutorial, we are going to learn how to get the style of current selection of Text using FabricJS. We can display text on canvas by adding an instance of fabric.Text. Not only does it allow us to move, scale and change the dimensions of the text but it also provides additional functionality like text alignment, text decoration, line height which can be obtained by the properties textAlign, underline and lineHeight respectively. We can also find the style of current selection by using the getSelectionStyles method.
Syntax
getSelectionStyles(startIndexopt, endIndexopt, completeopt)
Parameters
startIndexopt − This parameter accepts a Number which denotes the start index to get the styles at.
endIndexopt − This parameter accepts a Number which denotes the end index to get the styles at.
completeopt − This parameter accepts a Boolean value which decides whether to get full styles or not.
Example 1
Using the getSelectionStyles method
Let’s see a code example to see the logged output when the getSelectionStyles method is used. In this case, the styles from the 0th index all the way to the 4th index will be displayed.
<!DOCTYPE html> <html> <head> <!-- Adding the Fabric JS Library--> <script src="https://cdnjs.cloudflare.com/ajax/libs/fabric.js/510/fabric.min.js"></script> </head> <body> <h2>Using the getSelectionStyles method</h2> <p>You can open console from dev tools and see that the value is being displayed in the console</p> <canvas id="canvas"></canvas> <script> // Initiate a canvas instance var canvas = new fabric.Canvas("canvas"); canvas.setWidth(document.body.scrollWidth); canvas.setHeight(250); // Initiate a text object var text = new fabric.Text("Add sample
text here", { width: 300, fill: "green", fontWeight: "bold", }); // Add it to the canvas canvas.add(text); // Using getSelectionStyles method console.log("The style is", text.getSelectionStyles(0, 5, true)); </script> </body> </html>
Example 2
Using the getSelectionStyles method and passing different values
Let’s see a code example to see the logged output when the getSelectionStyles method is passed different values. In this case the logged output will contain the styles of the characters at 4th and 5th indices.
<!DOCTYPE html> <html> <head> <!-- Adding the Fabric JS Library--> <script src="https://cdnjs.cloudflare.com/ajax/libs/fabric.js/510/fabric.min.js"></script> </head> <body> <h2>Using the getSelectionStyles method and passing different values</h2> <p>You can open console from dev tools and see that the value is being displayed in the console</p> <canvas id="canvas"></canvas> <script> // Initiate a canvas instance var canvas = new fabric.Canvas("canvas"); canvas.setWidth(document.body.scrollWidth); canvas.setHeight(250); // Initiate a text object var text = new fabric.Text("Add sample
text here", { width: 300, fill: "green", fontWeight: "bold", styles: { 0: { 5: { fontSize: 55, fill: "blue", fontStyle: "oblique", }, 4: { fontSize: 45, fill: "pink", fontWeight: "bold", }, }, }, }); // Add it to the canvas canvas.add(text); // Using getSelectionStyles method console.log("The style is", text.getSelectionStyles(4, 6, true)); </script> </body> </html>