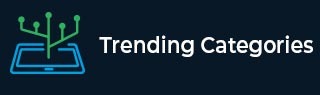
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to get the first element of the List in Java?
The List interface extends Collection interface. It is a collection that stores a sequence of elements. ArrayList is the most popular implementation of the List interface. User of a list has quite precise control over where an element to be inserted in the List. These elements are accessible by their index and are searchable.
List interface provides a get() method to get the element at particular index. You can specify index as 0 to get the first element of the List. In this article, we're exploring get() method usage via multiple examples.
Syntax
E get(int index)
Returns the element at the specified position.
Parameters
index - index of the element to return.
Returns
The element at the specified position.
Throws
IndexOutOfBoundsException - If the index is out of range (index < 0 || index >= size())
Example 1
Following is the example showing how to get the first element from a List.
package com.tutorialspoint; import java.util.ArrayList; import java.util.Arrays; import java.util.List; public class CollectionsDemo { public static void main(String[] args) { List<Integer> list = new ArrayList<>(Arrays.asList(4,5,6)); System.out.println("List: " + list); // First element of the List System.out.println("First element of the List: " + list.get(0)); } }
Output
This will produce the following result −
List: [4, 5, 6] First element of the List: 4
Example 2
Following is the example where getting the first element from a List can throw an exception.
package com.tutorialspoint; import java.util.ArrayList; import java.util.List; public class CollectionsDemo { public static void main(String[] args) { List<Integer> list = new ArrayList<>(); System.out.println("List: " + list); try { // First element of the List System.out.println("First element of the List: " + list.get(0)); } catch(Exception e) { e.printStackTrace(); } } }
Output
This will produce the following result −
List: [] java.lang.IndexOutOfBoundsException: Index: 0, Size: 0 at java.util.ArrayList.rangeCheck(ArrayList.java:659) at java.util.ArrayList.get(ArrayList.java:435) at com.tutorialspoint.CollectionsDemo.main(CollectionsDemo.java:11)