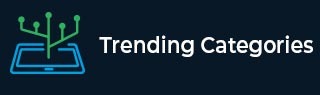
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to get the build/version number of an iOS App?
In this post we will learn how to fetch and show the iOS build and version number
Step 1 − Open Xcode → New Project → Single View Application → Let’s name it “ShowBuildAndVersion”
Step 2 − Open Main.storyboard and add two labels as shown below.
Step 3 − Attach @IBOutLets for the two labels
@IBOutlet weak var buildLabel: UILabel! @IBOutlet weak var versionLabel: UILabel!
Step 4 − Change the build and version from project settings.
Step 5 − In viewDidLoad of ViewController get the build and version number for infoDictionary of main bundle. Show it on the corresponding labels.
override func viewDidLoad() { super.viewDidLoad() if let version = Bundle.main.infoDictionary?["CFBundleShortVersionString"] as? String { versionLabel.text = "Version: \(version)" } if let build = Bundle.main.infoDictionary?["CFBundleVersion"] as? String { buildLabel.text = "Build: \(build)" } }
The infoDictionary in main bundle contains these values, with ‘CFBundleShortVersionString’ and ‘CFBundleVersion’ keys.
We can refer these keys to get the version and build number respectively
Step 6 − Run the project, you should see the build and version number.