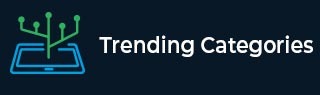
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to get a string from a tkinter filedialog in Python 3?
To interact with the filesystem in a tkinter application, you can use the Tkinter filedialog module. It provides a way to deal with the files in the system. The filedialog module offers many built-in functions to help developers create a variety of file dialogs for the application. You can use any of the filedialog functions in order to implement a dialog in your application.
The most commonly used function is filedialog.askopenfilename() which generally creates a dialog asking the user to open a file in the given program interface.
Example
Suppose we want to get a string or the filename which we open using the filedialog function. We can use the Label widget to display the filename we will open using the function. The following application can be used to open any type of file.
# Import required libraries from tkinter import * from tkinter import filedialog # Create an instance of tkinter window win = Tk() win.geometry("700x300") # Create a dialog using filedialog function win.filename=filedialog.askopenfilename(initialdir="C:/", title="Select a file") # Create a label widget label=Label(win, text="The File you have selected is: " + win.filename, font='Courier 11 bold') label.pack() win.mainloop()
Output
Running the above code will display a dialog asking the user to select a file from the C Drive.
Upon selecting a file, it will display the filepath on the window.