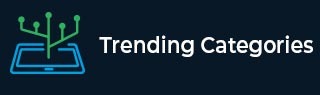
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to find the original size of an Image using FabricJS?
In this tutorial, we are going to learn how to find the original size of an Image using FabricJS. We can create an Image object by creating an instance of fabric.Image. Since it is one of the basic elements of FabricJS, we can also easily customize it by applying properties like angle, opacity etc. In order to find the original size of an Image, we use the getOriginalSize method.
Syntax
getOriginalSize(): Object
Using the getOriginalSize metho
Example
In this example, we have used the getOriginalSize method to obtain the width and height values of the image. Here, the width and height values being returned are 311 and 82 respectively.
<!DOCTYPE html> <html> <head> <!-- Adding the Fabric JS Library--> <script src="https://cdnjs.cloudflare.com/ajax/libs/fabric.js/510/fabric.min.js"></script> </head> <body> <h2>Using the getOriginalSize method</h2> <p> You can open the console from dev tools to see that the logged output contains the height and width of the Image </p> <canvas id="canvas"></canvas> <img src="https://www.tutorialspoint.com/images/logo.png" id="img1" style="display: none" /> <script> // Initiate a canvas instance var canvas = new fabric.Canvas("canvas"); canvas.setWidth(document.body.scrollWidth); canvas.setHeight(250); // Initiating the image element var imageElement = document.getElementById("img1"); // Initiate an Image object var image = new fabric.Image(imageElement, { top: 50, left: 110, skewX: 15, }); // Add it to the canvas canvas.add(image); // Using the getOriginalSize method console.log( "The original size of the Image object is: ", image.getOriginalSize() ); </script> </body> </html>
Using the getOriginalSize method along with cropX property
Example
Let’s see a code example of the logged output when the getOriginalSize method is used in conjunction with the cropX property. Here, we have passed a value of 50 to cropX. Due to this, our Image object will have a 50px crop from the original image size in the xdirection. However, when we use the getOriginalSize method we are returned the width and height values as 311 and 82 respectively which goes on to prove that getOriginalSize will only return the original size of the image.
<!DOCTYPE html> <html> <head> <!-- Adding the Fabric JS Library--> <script src="https://cdnjs.cloudflare.com/ajax/libs/fabric.js/510/fabric.min.js"></script> </head> <body> <h2>Using the getOriginalSize method along with cropX property</h2> <p> You can open the console from dev tools to see that the original size of the image will be returned regardless of having applied image cropping in xdirection </p> <canvas id="canvas"></canvas> <img src="https://www.tutorialspoint.com/images/logo.png" id="img1" style="display: none" /> <script> // Initiate a canvas instance var canvas = new fabric.Canvas("canvas"); canvas.setWidth(document.body.scrollWidth); canvas.setHeight(250); // Initiating the image element var imageElement = document.getElementById("img1"); // Initiate an Image object var image = new fabric.Image(imageElement, { top: 50, left: 110, skewX: 15, cropX: 50, }); // Add it to the canvas canvas.add(image); // Using the getOriginalSize method console.log( "The original size of the Image object is: ", image.getOriginalSize() ); </script> </body> </html>