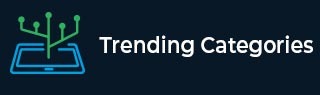
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to find a group of three elements in an array whose sum equals some target sum JavaScript
We have to write a function, say threeSum() that takes in an array of Numbers and a target sum. It checks whether there exist any three numbers in the array that add up to the target sum, if there exist such three numbers in the array, then it should return their indices in an array otherwise it should return -1.
Approach
The approach is simple, We will first write a function twoSum(), that takes in an array and a target sum and takes linear time and space to return the indices of two numbers that add up to target sum otherwise -1.
Then we write the actual function threeSum(), that iterates over each element in the array to find the index of the third element which when added to the twoSum() numbers can add up to the actual target.
Therefore, like this we can find the three elements in O(N^2) time. Let’s write the code for this −
Example
const arr = [1,2,3,4,5,6,7,8]; const twoSum = (arr, sum) => { const map = {}; for(let i = 0; i < arr.length; i++){ if(map[sum-arr[i]]){ return [map[sum-arr[i]], i]; }; map[arr[i]] = i; }; return -1; }; const threeSum = (arr, sum) => { for(let i = 0; i < arr.length; i++){ const indices = twoSum(arr, sum-arr[i]); if(indices !== -1 && !indices.includes(i)){ return [i, ...indices]; }; }; return -1; }; console.log(threeSum(arr, 9)); console.log(threeSum(arr, 8)); console.log(threeSum(arr, 13)); console.log(threeSum(arr, 23));
Output
The output in the console will be −
[ 0, 2, 4 ] [ 0, 2, 3 ] [ 0, 4, 6 ] -1