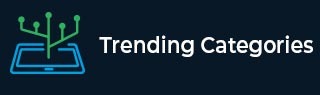
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to fetch an Integer variable as String in GoLang?
In GoLang, converting an integer variable to a string is a common requirement when dealing with different data types. Luckily, GoLang provides several built-in methods that make this conversion easy. In this article, we'll explore how to fetch an integer variable as a string in GoLang.
Step 1: Declare the Integer Variable
First, let's declare an integer variable. We will use this variable in subsequent steps to convert it into a string.
num := 100
Step 2: Convert Integer to String using strconv.Itoa()
To convert the integer variable to a string, we will use the strconv package. The strconv package provides the Itoa() function, which takes an integer value and returns its corresponding string representation.
import "strconv" numStr := strconv.Itoa(num)
The Itoa() function takes an integer argument and returns a string representation of that integer.
Step 3: Print the Converted String
Now that we have the integer converted to a string, we can print it to the console or use it in any other context where a string is required.
fmt.Println("Number as String: " + numStr)
This code will print the following output −
Number as String: 100
Example
package main import ( "fmt" "strconv" ) func main() { num := 42 str := strconv.Itoa(num) fmt.Printf("The integer value is %d and the string value is %s", num, str) }
Output
The integer value is 42 and the string value is 42
Conclusion
In this article, we saw how to fetch an integer variable as a string in GoLang using the strconv package's Itoa() function. Converting between data types is a common requirement in GoLang, and knowing how to perform these conversions is essential to writing effective GoLang code.