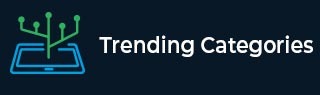
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to encode and decode a URL in JavaScript?
Encoding and decoding the URI and the URI components is required by the URL of any website to reach or redirect the user. It is a usual task in web development, and this is generally done while making a GET request to the API with the query params. The query params must also be encoded in the URL string, where the server will decode this. Many browsers automatically encode and decode the URL and the response string.
E.g., A space " " is encoded as a + or %20.
Encoding a URL
The conversion of the special characters can be done by using the following method from JavaScript −
encodeURI() function − The encodeURI() function is used for encoding the complete URI, i.e., converting the special characters from the URI into browser understandable language. Some of the characters that are not encoded are: (, / ? : @ & = + $ #).
encodeURIComponent() function − This function encodes the whole URL instead of just the URI. The component encodes the domain name also.
Syntax
encodeURI(complete_uri_string ) encodeURIComponent(complete_url_string )
Parameter
complete_uri_string string − It holds the URL to be encoded.
complete_url_string string − It holds the complete URL string to be encoded.
The above functions return the encoded URL.
Example 1
In the below example, we encode a URL using encodeURI() and encodeURIComponent() methods.
# index.html
<!DOCTYPE html> <html lang="en"> <head> <title>Encoding URI</title> </head> <body> <h1 style="color: green;"> Welcome To Tutorials Point </h1> <script> const url="https://www.tutorialspoint.com/search?q=java articles"; document.write('<h4>URL: </h4>' + url) const encodedURI=encodeURI(url); document.write('<h4>Encoded URL: </h4>' + encodedURI) const encodedURLComponent=encodeURIComponent(url); document.write('<h4>Encoded URL Component: </h4>' + encodedURLComponent) </script> </body> </html>
Output
Decoding a URL
The decoding of a URL can be done using the following methods −
decodeURI() function − The decodeURI() function is used to decode the URI, i.e., converting the special characters back to the original URI language.
decodeURIComponent() function − This function decodes the complete URL back to its original form. The decodeURI only decodes the URI part, whereas this method decodes the URL, including the domain name.
Syntax
decodeURI(encoded_URI ) decodeURIComponent(encoded_URL
Parameter
encoded_URI URI − It takes input for the encoded URL created by the encodeURI() function.
encoded_URL URL − It takes input for the encoded URL created by the encodeURIComponent() function.
These functions will return the decoded format of the encoded URL.
Example 2
In the below example, we decode an encoded URL using decodeURI() and decodeURIComponent() methods into its original form.
# index.html
<!DOCTYPE html> <html lang="en"> <head> <title>Encode & Decode URL</title> </head> <body> <h1 style="color: green;"> Welcome To Tutorials Point </h1> <script> const url="https://www.tutorialspoint.com/search?q=java articles"; const encodedURI = encodeURI(url); document.write('<h4>Encoded URL: </h4>' + encodedURI) const encodedURLComponent = encodeURIComponent(url); document.write('<h4>Encoded URL Component: </h4>' + encodedURLComponent) const decodedURI=decodeURI(encodedURI); document.write('<h4>Decoded URL: </h4>' + decodedURI) const decodedURLComponent = decodeURIComponent(encodedURLComponent); document.write('<h4>Decoded URL Component: </h4>' + decodedURLComponent) </script> </body> </html>