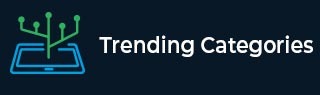
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to delete a single record in Laravel 5?
There are different ways you can do it. Let us test a few examples that help us delete a single record in Laravel.
Assume we have created a table named users table as shown below −
mysql> select * from users; +----+---------------+------------------+--------------+------------+------+ | id | name | email | password | address | age | +----+---------------+------------------+--------------+------------+------+ | 1 | Siya Khan | siya@gmail.com | hgfdv3vhjd | Xyz | 20 | | 2 | Rehan Khan | rehan@gmail.com | cskjg367dv | Xyz | 18 | | 3 | Rehan Khan | rehan@gmail.com | dshjgcv2373h | testing | 20 | | 4 | Rehan | rehan@gmail.com | abcd | vci4hn4f4 | 15 | | 5 | Nidhi Agarwal | nidhi@gmail.com | fjh99302v | abcd | 20 | | 6 | Ashvik Khanna | ashvik@gmail.com | 83bg44f4f | oooo | 16 | | 7 | Viraj Desai | viraj@gmail.com | ppsdkj39hgb2 | test | 18 | | 8 | Priya Singh | priya@gmail.com | chjbbvgy3ij | test123 | 20 | +----+---------------+------------------+--------------+------------+------+
Example 1
You can use find() method accepts a key value as a parameter and returns the model that has the given key as the primary key. The delete() method removes the current record. Following example demonstrates how to remove a record using these two methods
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Models\User; class UserController extends Controller { public function index() { $userDetails = User::find(9); $deleteuser = $userDetails->delete(); if ($deleteuser) { echo "Record deleted from table successfully"; } else { echo "Error in Deletion"; } } }
Output
The output of the above code is as follows
Record deleted from table successfully
Example 2
You can add condition in where clause to delete the record from table as shown below −
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Models\User; class UserController extends Controller{ public function index() { $deleteuser = User::where('id', '=', 11)->delete(); if ($deleteuser) { echo "Record deleted from table successfully"; } else { echo "Error in Deletion"; } } }
Output
The output of the above code is −
Record deleted from table successfully
Example 3
You can also make use of the destroy() method to delete records from the table.
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Models\User; class UserController extends Controller { public function index() { $deleteuser = User::destroy(12);; if ($deleteuser) { echo "Record deleted from table successfully"; } else { echo "Error in Deletion"; } } }
Output
The output of the above code is −
Record deleted from table successfully
Example 4
If you want to delete multiple records at a time you can do so as shown below −
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Models\User; class UserController extends Controller{ public function index() { $deleteuser = User::destroy([15,16,17]); if ($deleteuser) { echo "Record deleted from table successfully"; } else { echo "Error in Deletion"; } } }
Output
The output of the above code is −
Record deleted from table successfully