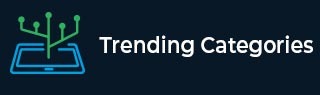
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to create the previous and next buttons and non-working on the end positions using JavaScript?
We can create previous and next buttons that would be non-working (or disabled) at their end positions by using vanilla javascript. Javascript is a powerful browser-level language with which we can control and manipulate the DOM elements easily. Here, we will create 2 buttons, and change an HTML element’s content depending on which button is clicked.
Example 1
In this example, we will create an “increment” and a “decrement” button which, when clicked, will increment and decrement the HTML element’s content value by 1, respectively. We will also disable the buttons when they reach their extreme positions, which will be 5 for the increment button and 0 for the decrement button.
<html lang="en"> <head> <title>How to create previous and next button and non-working on end position using JavaScript?</title> </head> <body> <h3>How to create previous and next button and non-working on end position using JavaScript?</h3> <p>3</p> <div> <button class="inc">Increment</button> <button class="dec">Decrement</button> </div> <script> const inc = document.getElementsByClassName('inc')[0] const dec = document.getElementsByClassName('dec')[0] const content = document.getElementsByTagName('p')[0] let i = 3; inc.addEventListener('click', () => { if (i === 5) return; i++; content.textContent = i; }) dec.addEventListener('click', () => { if (i === 0) return; i--; content.textContent = i; }) </script> </body> </html>
Example 2
In this example, let’s implement the above approach, but instead of showing the increase and decrease in number, let’s show the increase and decrease in the left alignment of an “absolute” positioned element.
<html lang="en"> <head> <title>How to create previous and next button and non-working on end position using JavaScript?</title> <style> body { position: relative; } .dot { background-color: black; height: 5px; width: 5px; border-radius: 50%; position: absolute; } .inc, .dec { margin-top: 20px; } </style> </head> <body> <h3>How to create previous and next button and non-working on end position using JavaScript?</h3> <div class="dot"></div> <div> <button class="inc">Increment</button> <button class="dec">Decrement</button> </div> <script> const inc = document.getElementsByClassName('inc')[0] const dec = document.getElementsByClassName('dec')[0] const dot = document.getElementsByClassName('dot')[0] let i = 0; inc.addEventListener('click', () => { if (i === 10) return; i += 2; dot.style.left = `${i}%` }) dec.addEventListener('click', () => { if (i === 0) return; i -= 2; dot.style.left = `${i}%` }) </script> </body> </html>
Conclusion
In this article, we learned how to create a paginated layout in a web application using Bootstrap 4, using two different examples. In the first example, we displayed the increment and decrement using a number, and in the second example, we displayed the increment and decrement of a value using the left value of an “absolute” positioned element.