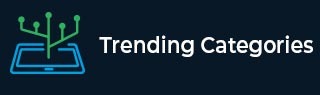
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to create regular expression only accept special formula?
The regular expression is a pattern containing various characters. We can use the regular expression to search whether a string contains a particular pattern.
Here, we will learn to create a regular expression to validate the various mathematical formulas. We will use the test() or match() method to check if particular mathematical formula matches with regular expression or not
Syntax
Users can follow the syntax below to create regular expressions accepting special mathematical formulas.
let regex = /^\d+([-+]\d+)*$/g;
The above regex accepts only 10 – 13 + 12 + 23, like mathematical formulas.
Regular Expression Explanation
/ / – It represents the start and end of the regular expression.
^ – It represents the start of the formula string.
\d+ – It represents at least one or more digits at the start of the formula.
[-+] – It represents the ‘+’ and ‘-‘ operators in regular expression.
([-+]\d+)* – It represents that formula can contain digits followed by ‘+’ or ‘-‘ operator multiple times.
$ – It represents the end of the string.
g – It is an identifier to match all occurrences.
Example
In the example below, we have created the regular expression which accepts the formula containing ‘+’ or ‘-‘ operators with digits.
Users can observe that the first formula matches the regex pattern in the output. The second formula is not matching with regex pattern as it contains the ‘*’ operator. Also, the third formula is the same as the first, but it contains the space between the operator and digit, so it doesn’t match with regular expressions.
<html> <body> <h3>Creating the regular expression to validate special mathematical formula in JavaScript</h3> <div id = "output"></div> <script> let output = document.getElementById('output'); function matchFormula(formula) { let regex = /^\d+([-+]\d+)*$/g; let isMatch = regex.test(formula); if (isMatch) { output.innerHTML += "The " + formula + " is matching with " + regex + "<br>"; } else { output.innerHTML += "The " + formula + " is not matching with " + regex + "<br>"; } } let formula = "10+20-30-50"; matchFormula(formula); matchFormula("60*70*80"); matchFormula("10 + 20 - 30 - 50") </script> </body> </html>
A regular expression used in the example below
We have used the /^\d+(\s*[-+*/]\s*\d+)*$/g regular expression in the below example. Users can find the explanation of the used regular expression below.
^\d+ – It represents at least one digit at the start of the formula.
\s* – It represents zero or more white spaces.
(\s*[-+*/]\s*\d+)* – It represents that formula can contain space, operator, space, and digits multiple times in the same order.
Example
In the example below, we have invoked the TestMultiplyFormula() function three times by passing various formulas as a parameter. We have used the test() method to check if the formula matches the regular expression pattern.
In the output, we can see that regular expression accepts the formulas with the ‘*’ and ‘/’ operators and white spaces.
<html> <body> <h2>Creating the regular expression <i> to validate special mathematical formula </i> in JavaScript.</h2> <div id = "output"> </div> <script> let output = document.getElementById('output'); function TestMultiplyFormula(formula) { let regex = /^\d+(\s*[-+*/]\s*\d+)*$/g; let isMatch = regex.test(formula); if (isMatch) { output.innerHTML += "The " + formula + " is matching with " + regex + "<br>"; } else { output.innerHTML += "The " + formula + " is not matching with " + regex + "<br>"; } } let formula = "12312323+454+ 565 - 09 * 23"; TestMultiplyFormula(formula); TestMultiplyFormula("41*14* 90 *80* 70 + 90"); TestMultiplyFormula("41*14& 90 ^80* 70 + 90"); </script> </body> </html>
This tutorial taught us to create a regular expression that accepts special mathematical formulas. In both examples, we have used the test() method to match the formula with regular expression. Also, we have used different regular expression patterns in both examples.