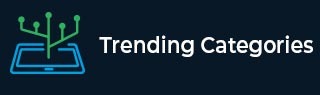
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to create Java Priority Queue to ignore duplicates?
The simplest way to create a Java Priority Queue to ignore duplicates is to first create a Set implementation −
HashSet <Integer> set = new HashSet <> (); set.add(100); set.add(150); set.add(250); set.add(300); set.add(250); set.add(500); set.add(600); set.add(500); set.add(900);
Now, create Priority Queue and include the set to remove duplicates in the above set −
PriorityQueue<Integer>queue = new PriorityQueue<>(set);
Example
import java.util.HashSet; import java.util.PriorityQueue; public class Demo { public static void main(String[] args) { HashSet<Integer>set = new HashSet<>(); set.add(100); set.add(150); set.add(250); set.add(300); set.add(250); set.add(500); set.add(600); set.add(500); set.add(900); PriorityQueue<Integer>queue = new PriorityQueue<>(set); System.out.println("Elements with no duplicates = "+queue); } }
Output
Elements with no duplicates = [100, 150, 250, 500, 600, 900, 300]
Advertisements