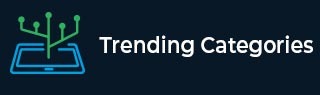
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to create Frame by Frame Animation using CSS and JavaScript?
Frame by frame animation is a technique used in animation to produce motion by displaying a series of static images which are sequentially displayed. The appearance of motion is achieved by displaying the images in rapid succession.
The followings are needed before we go to create the frame by frame animation−
A series of images (frames)
A web page with CSS and JavaScript
Approach
The process of creating frame by frame animation using CSS and JavaScript is relatively simple.
STEP 1 – First, you need to create a series of images (frames) that you want to be displayed in succession.
STEP 1 – Next, you need to create a web page with CSS and JavaScript that will load and display the images in rapid succession.
Full working code example
Here is a full working code example of how to create frame by frame animation using CSS and JavaScript. The code will load and display 2 images in succession.
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>Frame by Frame Animation</title> <style> #container { width: 400px; height: 400px; position: relative; } #container img { position: absolute; top: 0; left: 0; } </style> </head> <body> <div id="container"> <img src="https://www.tutorialspoint.com/static/images/logo-color.png" /> <img src="https://www.tutorialspoint.com/images/QAicon-black.png" /> </div> <script> var container = document.getElementById('container'); var images = container.getElementsByTagName('img'); var currentImage = 0; function changeImage() { images[currentImage].style.display = 'none'; currentImage = (currentImage + 1) % images.length; images[currentImage].style.display = 'block'; } setInterval(changeImage, 1000); </script> </body> </head>
HTML
The HTML code is very simple. It consists of a div element with an id of "container". Within the div element, there are 2 img elements. These img elements are the frames of the animation.
CSS
The CSS code styles the div element and the img elements. The div element is given a width and height. The img elements are positioned absolutely within the div element.
JavaScript
The JavaScript code is where the magic happens. First, the code gets a reference to the div element and the img elements. Next, the code defines a variable named "currentImage". This variable will be used to keep track of which image is currently being displayed.
The code then defines a function named "changeImage". This function will hide the current image and display the next image in the sequence.
Finally, the code uses the setInterval function to call the "changeImage" function every 1000 milliseconds (1 second). This will cause the images to be displayed in rapid succession, creating the illusion of motion.
And that's all there is to it! With just a few lines of code, you can create a simple CSS and JavaScript frame by frame animation.