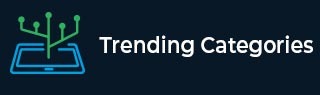
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to create an off-canvas menu with CSS and JavaScript?
In this, article we are going to create off canvas menu using CSS and JavaScript.
Off−canvas menu lets you display your content as a sidebar when a button is activated. This helps you to display a certain aspect of your site in the side navigation panel thus giving a user−friendly familiarity to your guests.
An off−canvas menu can also be accessed by sliding left−to−right or right−to−left (if you are using a touchscreen device) depending on the design of your website. This is usually used in cases where a website has a lot of links that don’t fit in the top horizontal navigation bar.
In the following example, we are doing off canvas menu, if you click the menu bar then the navigation will be visible and page content will be hidden.
Example.html
Create an HTML file in which we will define the structure (view) of the page. In this example Using the HTML code we are creating the current page with required text, a side bar overlay menu and empty navigation Links for the menu.
<body> <div id="mySide-nav" class="side-nav"> <a href="javascript:void(0)" class="closebtn" onclick="closeNav()">×</a> <a href="#">About</a> <a href="#">Services</a> <a href="#">Clients</a> <a href="#">Contact</a> </div> <div id="main"> <span style="font-size: 30px; cursor: pointer" onclick="openNav()">☰</span> <h2>Side Navigation bar</h2> <p>click on the above menu bar to see how off-canvas menu work.</p> </div>
Example.css
Add CSS style to give some animation effect to web page elements for a better look. In this example we are styling the side navigation bar (adding the color, specifying the width of the navigation bar) and adding hover effect of the elements of the menu (red color).
<style> body { font-family: Georgia, "Times New Roman", Times, serif; } .side-nav { height: 100%; width: 0; position: fixed; z-index: 1; top: 0; left: 0; background-color: rgb(60, 198, 136); overflow-x: hidden; transition: 0.5s; padding-top: 60px; } .side-nav a { padding: 8px 8px 8px 32px; text-decoration: none; font-size: 20px; color: white; display: block; transition: 0.3s; font-weight: bold; font-style: italic; } .side-nav a:hover { color: red; } .side-nav .closebtn { position: absolute; top: 0; right: 25px; font-size: 36px; margin-left: 50px; } #main { transition: margin-left 0.5s; padding: 16px; } #main span:hover { color: green; } @media screen and (max-height: 450px) { .side-nav { padding-top: 15px; } .side-nav a { font-size: 18px; } } </style>
Example.js
Using JavaScript, we can perform validation and handle events on a page. In this example we will use Java Script code to open and close the side bar menu.
For this purpose, we can use listeners which will capture (listen) the mouse−click actions on the menu and the close button and perform the respective actions.
<script> function openNav() { document.getElementById("mySide-nav").style.width = "250px"; document.getElementById("main").style.display = "none"; } function closeNav() { document.getElementById("mySide-nav").style.width = "0"; document.getElementById("main").style.display = "block"; } </script>
Complete Example
<!DOCTYPE html> <html> <head> <meta name="viewport" content="width=device-width, initial-scale=1" /> <style> body { font-family: Georgia, "Times New Roman", Times, serif; } .side-nav { height: 100%; width: 0; position: fixed; z-index: 1; top: 0; left: 0; background-color: rgb(60, 198, 136); overflow-x: hidden; transition: 0.5s; padding-top: 60px; } .side-nav a { padding: 8px 8px 8px 32px; text-decoration: none; font-size: 20px; color: white; display: block; transition: 0.3s; font-weight: bold; font-style: italic; } .side-nav a:hover { color: red; } .side-nav .closebtn { position: absolute; top: 0; right: 25px; font-size: 36px; margin-left: 50px; } #main { transition: margin-left 0.5s; padding: 16px; } #main span:hover { color: green; } @media screen and (max-height: 450px) { .side-nav { padding-top: 15px; } .side-nav a { font-size: 18px; } } </style> </head> <body> <div id="mySide-nav" class="side-nav"> <a href="javascript:void(0)" class="closebtn" onclick="closeNav()">×</a> <a href="#">About</a> <a href="#">Services</a> <a href="#">Clients</a> <a href="#">Contact</a> </div> <div id="main"> <span style="font-size: 30px; cursor: pointer" onclick="openNav()">☰</span> <h2>Side Navigation bar</h2> <p>click on the above menu bar to see how off-canvas menu work.</p> </div> <script> function openNav() { document.getElementById("mySide-nav").style.width = "250px"; document.getElementById("main").style.display = "none"; } function closeNav() { document.getElementById("mySide-nav").style.width = "0"; document.getElementById("main").style.display = "block"; } </script> </body> </html>