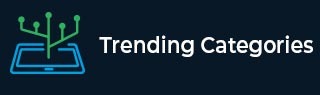
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to create an instance of a Metaclass that run on both Python2 and Python3?
Metaclasses are a concept in object-oriented programming where a class is an instance of another class, known as the metaclass. They allow for the customization of class creation and behaviour, enabling the creation of classes with specific attributes and methods. A metaclass is the blueprint of the class itself, just like a class is the blueprint for instances of that class. They can be used to enforce coding standards, create automatic APIs, or perform other advanced tasks that are impossible with standard inheritance.
Python supports metaclasses, which create custom classes with unique behaviour. Metaclasses can also add special methods or attributes to a class or modify its definition, which can be useful in situations where specific behaviour needs to be added to every instance of a certain class.
We will illustrate two examples to create a metaclass that runs both on Python2 and Python3. By the end, we can create metaclass using two different methods using ‘type()’ and ‘six.with_metaclass()’.
Syntax
class Mynewclass(type): def __init__(cls, name, bases, dict): pass
In this syntax, we used ‘__init__’ to initialize the created object passed as a parameter. ‘name’ represents the name of the class, whereas ‘bases’ defines a tuple of bases class from which the class will inherit and ‘dict’ defines namespace dictionary which contains the definition of class.
Example 1
Here’s an example to create a metaclass using the ‘six.with_metaclass()’ function, which is the built-in function used to create new classes in Python. In this example, we initialized the class using the ‘__new__’ method, and we have created a class by providing the MyMClass class to the metaclass keyword argument.
from six import with_metaclass class MyMetaClass(type): def __new__(cls, name, bases, attrs): return super(MyMetaClass, cls).__new__(cls, name, bases, attrs) class MyClass(with_metaclass(MyMetaClass)): pass print(type(MyClass))
Output
<class '__main__.MyMetaClass'>
Example 2
In this example, we used the ‘__new__’ method of the metaclass; we can use the six.PY2 variable to check which version of Python is being used and handle any differences accordingly. Then, we printed the Python version and called the super method to create the class using the standard type behaviour. By using the six modules and checking for Python version-specific syntax and behaviour, we can create a metaclass that works on both Python 2 and Python 3.
import six class MyMetaClass(type): def __new__(cls, name, bases, attrs): if six.PY2: print("This is python2") return super(MyMetaClass, cls).__new__(cls, name, bases, attrs) else: print("This is python3") return super(MyMetaClass, cls).__new__(cls, name, bases, attrs) class MyClass(six.with_metaclass(MyMetaClass)): pass print(type(MyClass))
Output
This is python3 <class '__main__.MyMetaClass'>
Conclusion
We learned that Metaclasses are a powerful tool in Python that allows for the customization of class creation and behaviour. Also, Python 2 and Python 3 have different syntaxes for creating and using metaclasses, but there are ways to create metaclasses that work on both versions. The ‘six’ library provides a ‘with_metaclass()’ helper function that can be used to create metaclasses that work on both versions of Python.
Metaclasses are a powerful feature of Python, but they are also an advanced topic that might not be necessary for all projects. One use case for metaclasses is creating domain-specific languages (DSLs) tailored to a specific problem domain. For example, a metaclass could be used to create a DSL for describing user interfaces, allowing developers to create UI components with a simple and intuitive syntax. Overall, metaclasses are a powerful tool that can be used to customize class behaviour and create advanced Python features.