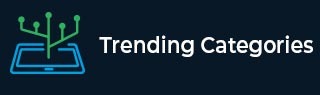
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to create an ember handlebars template?
Ember.js is a framework based on javascript that is widely used in building complex web applications. The framework allows developers to create single-page web applications that are scalable just by using some common idioms, best practices, and patterns from other single-page-app ecosystem patterns in the framework.
Handlebars templating system is one of its key features that provides a simple and powerful way to create dynamic web pages. In this article, we will see how to create an ember handlebars template.
What is Template in Ember?
Templates in ember are used in defining the user interface (UI) of a web application. Templates are written using the handlebars syntax, which is a simple templating language used to create dynamic HTML pages by embedding data in HTML markup.
Ember js templates use a combination of HTML & handlebars syntax to create a user interface that is used to respond to changes in data. This includes logic such as conditionals, loops, and computed properties allowing the developers to create complex and dynamic UIs with less code.
They are structured as a hierarchy of components where each component represents a specific part of the UI. The component may include other components that are used to allow for complex and nested structures. The rendered component generates HTML based on its template and any data or parameters that are passed to it.
What is Handlebar Template in Ember?
Handlebars is a popular templating language for creating dynamic HTML pages. It provides a simple syntax for embedding data into HTML markup, allowing developers to create dynamic and responsive user interfaces with minimal code.
In Handlebars, templates are defined using a combination of HTML markup and Handlebars expressions. Handlebars expressions are enclosed in double curly braces ({{ }}) and can be used to display data, iterate over lists, and conditionally display content.
<ul> {{#each items as |item|}} <li>{{item}}</li> {{/each}} </ul>
In this template, the {{#each}} expression is used to iterate over a list of items, and the {{item}} expression is used to display each item in a list item (<li>).
Expressions in the Handlebar Template
Expressions are used to embed dynamic content into the HTML. They are enclosed within curly braces {{}} and may contain variables, helper functions, and conditional statements.
Let’s say, we have a variable named last-name containing a string value "World" and we want to display it in our Handlebars template, then we can use the following expression −
<h1>Hello, {{last-name}}!</h1>
Steps to Create an Ember Handlebar Template
To create an ember handlebar template, there are some steps that need to follow. Let’s see the steps one by one in detail.
Step 1: Create an Ember Project
The first step in creating an Ember Handlebars template is to set up an Ember.js project. This can be done just by following the steps −
Install Ember
npm install -g ember-cli
Create a new application
ember new ember-quickstart --lang en
Run the server
cd ember-quickstart ember serve
Step 2: Create a New Handlebar Template
Once, you have created the ember project, now it's time to create a new handlebar template.
Note that the handlebars templates have a .hbs file extension, and are typically stored in the app/templates directory of your Ember.js project.
Now go to the app/templates and create a new Handlebars template file, simply create a new file with a .hbs file extension. Let’s say, we create a file called my-template.hbs.
Step 3: Define the Template
The next step is to define the handlebars template and add some content to it. As told earlier, handlebar templates use a simple syntax for defining dynamic content. Below is an example of a basic Handlebars template −
<div class="my-new-template"> <h1>{{firstname}}</h1> <p>{{lastname}}</p> </div>
In the above code, the Handlebars template is defined using a div element with a class of "my-new-template". Here, in the div, we have defined two dynamic elements with the help of the Handlebars syntax. The first is an h1 element with the text {{firstname}}, and the second is a p element with the text {{lastname}}.
If you don’t use build tools then you can simply define your application’s template inside your HTML using a script tag, see below −
<html> <body> <script type="text/x-handlebars"> <strong>{{firstname}}, {{lastname}}</strong>! </script> </body> </html>
Step 4: Using the Defined Template
Once you have defined your Handlebars template, you can use it in your Ember.js application. To do this, you will need to create a route and a corresponding controller that will render the template.
Here is an example of how to create a route and a controller that use the my-new-template Handlebars template −
// app/routes/my-route.js import Route from '@ember/routing/route'; export default class MyRoute extends Route { model() { return { firstname: ‘Hello’, lastname: ‘World’ }; } } // app/controllers/my-controller.js import Controller from '@ember/controller'; export default class MyController extends Controller { }
In this example, the MyRoute class defines a model method that returns an object with a title and body property. The MyController class is empty, but it will automatically render the my-new-template Handlebars template because its name matches the name of the route.
Step 5: Rendering the Template
The final step is to render the template in your application. To do this, you will need to add a template outlet to your application's main template file. Here is an example of how to do this −
<!-- app/templates/application.hbs --> <div class="container"> {{outlet}} </div>
In this example, the main application template file defines a div element with a class of "container". Inside the div, there is a template outlet, which will render the my-new-template Handlebars template when the MyRoute is active.
Output
Hello, World!
Conclusion
The Handlebars template is an important component of Ember.js that enables developers in developing a dynamic and adaptable UI with minimal code and effort. It uses a combination of HTML and Handlebars expressions to establish templates that can respond to alterations in data. In this article, we learned the steps to create an ember handlebars template. First, we set up an Ember project, then generated a new handlebar template, defined the template, use the defined template, and finally, rendered the template in our application. With Ember Handlebars templates, we can easily construct intricate and dynamic UIs for their web applications.