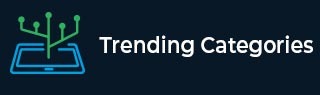
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to create a watermark on an image using OpenCV Python?
To add a watermark to an image, we will use the cv2.addWeighted() function from OpenCV. You can use the following steps to create a watermark on an input image −
Import the required library. In all the following Python examples, the required Python library is OpenCV. Make sure you have already installed it.
import cv2
Read the input image on which we are going to apply the watermark and read the watermark image.
img = cv2.imread("panda.jpg") wm = cv2.imread("watermark.jpg")
Access the height and width of the input image, and the height, width of the watermark image.
h_img, w_img = img.shape[:2] h_wm, w_wm = wm.shape[:2]
Calculate the coordinates of the center of the image. We are going to place the watermark at the center.
center_x = int(w_img/2) center_y = int(h_img/2)
Calculate roi from top, bottom, right and left.
top_y = center_y - int(h_wm/2) left_x = center_x - int(w_wm/2) bottom_y = top_y + h_wm right_x = left_x + w_wm
Add the watermark to the input image.
roi = img[top_y:bottom_y, left_x:right_x] result = cv2.addWeighted(roi, 1, wm, 0.3, 0) img[top_y:bottom_y, left_x:right_x] = result
Display the watermarked image. To display the image, we use cv2.imshow() function.
cv2.imshow("Watermarked Image", img) cv2.waitKey(0) cv2.destroyAllWindows()
Let's have a look at the example below for a better understanding.
We will use the following images as the Input Files in this program −
Example
In this Python program, we added a watermark to the input image.
# import required libraries import cv2 # Read the image on which we are going to apply watermark img = cv2.imread("panda.jpg") # Read the watermark image wm = cv2.imread("watermark.jpg") # height and width of the watermark image h_wm, w_wm = wm.shape[:2] # height and width of the image h_img, w_img = img.shape[:2] # calculate coordinates of center of image center_x = int(w_img/2) center_y = int(h_img/2) # calculate rio from top, bottom, right and left top_y = center_y - int(h_wm/2) left_x = center_x - int(w_wm/2) bottom_y = top_y + h_wm right_x = left_x + w_wm # add watermark to the image roi = img[top_y:bottom_y, left_x:right_x] result = cv2.addWeighted(roi, 1, wm, 0.3, 0) img[top_y:bottom_y, left_x:right_x] = result # display watermarked image cv2.imshow("Watermarked Image", img) cv2.waitKey(0) cv2.destroyAllWindows()
Output
On the execution of the above code, it will produce the following output window.