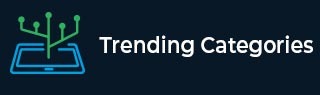
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to create a "user rating" scorecard with CSS?
To create a user rating scorecard, first set the exact icon for a star. That would be done using the Font Awesome icons. The individual ratings are displayed as progress bars.
Set the Icon for Star Rating
The icon for the star rating is set using Font Awesome Icons. We have added the following CDN path for the Font Awesome icons in the beginning to use it on our web page −
<link href="https://stackpath.bootstrapcdn.com/font-awesome/4.7.0/css/font-awesome.min.css" rel="stylesheet" integrity="sha384-wvfXpqpZZVQGK6TAh5PVlGOfQNHSoD2xbE+QkPxCAFlNEevoEH3Sl0sibVcOQVnN" crossorigin="anonymous" />
For rated, use the fa fa-star rated −
<span class="fa fa-star rated"></span> <span class="fa fa-star rated"></span> <span class="fa fa-star rated"></span> <span class="fa fa-star rated"></span>
The above rated class is styled to make the star look appealing −
.rated { color: rgb(255, 0, 0); border: 2px solid yellow; }
For non-rated, use only fa fa-star −
<span class="fa fa-star"></span>
The Star Rating Header
The star rating header is the following −
<span class="rateHeader">User Rating</span>
It is styled like this −
.rateHeader { font-size: 25px; margin-right: 25px; }
Set div for Progress bar
Different progress bars are created for 1star, 2 star, 3star, 4star, and 5star ratings −
<div class="row"> <div class="data"> <div>5 star</div> </div> <div class="progressBar"> <div class="progressContainer"> <div class="bar-5"></div> </div> </div> <div class="data right"> <div>120</div> </div> <div class="data"> <div>4 star</div> </div> <div class="progressBar"> <div class="progressContainer"> <div class="bar-4"></div> </div> </div> <div class="data right"> <div>110</div> </div> <div class="data"> <div>3 star</div> </div> <div class="progressBar"> <div class="progressContainer"> <div class="bar-3"></div> </div> </div> <div class="data right"> <div>30</div> </div> <div class="data"> <div>2 star</div> </div> <div class="progressBar"> <div class="progressContainer"> <div class="bar-2"></div> </div> </div> <div class="data right"> <div>20</div> </div> <div class="data"> <div>1 star</div> </div> <div class="progressBar"> <div class="progressContainer"> <div class="bar-1"></div> </div> </div> <div class="data right"> <div>12</div> </div> </div>
Style the Progress bar
The progress bar is placed on the left with the float property with the left value −
.progressBar { margin-top: 10px; float: left; width: 70%; }
Style the Progress bar Container
The container div in the progress bar is styled like this −
.progressContainer { width: 100%; background-color: #f1f1f1; text-align: center; color: white; border-radius: 10px; }
Style the Individual Bars
The individual bars are also styled with the width, height, and the background-color property −
.bar-5 { width: 70%; height: 18px; background-color: rgb(76, 175, 162); } .bar-4 { width: 50%; height: 18px; background-color: rgb(243, 222, 33); } .bar-3 { width: 20%; height: 18px; background-color: #12d400; } .bar-2 { width: 65%; height: 18px; background-color: #ff0055; } .bar-1 { width: 40%; height: 18px; background-color: #a836f4; }
Example
To create a “user rating” scorecard with CSS, the code is as follows −
<!DOCTYPE html> <html> <head> <link href="https://stackpath.bootstrapcdn.com/font-awesome/4.7.0/css/font-awesome.min.css" rel="stylesheet" integrity="sha384-wvfXpqpZZVQGK6TAh5PVlGOfQNHSoD2xbE+QkPxCAFlNEevoEH3Sl0sibVcOQVnN" crossorigin="anonymous" /> <style> * { box-sizing: border-box; } body { font-family: "Segoe UI", Tahoma, Geneva, Verdana, sans-serif; margin: 30px; max-width: 800px; padding: 20px; } .rateHeader { font-size: 25px; margin-right: 25px; } .fa { font-size: 25px; color: grey; } .rated { color: rgb(255, 0, 0); border: 2px solid yellow; } .data { float: left; width: 15%; margin-top: 10px; font-weight: bold; } .progressBar { margin-top: 10px; float: left; width: 70%; } .right { text-align: right; } .row:after { content: ""; display: table; clear: both; } .progressContainer { width: 100%; background-color: #f1f1f1; text-align: center; color: white; border-radius: 10px; } .bar-1, .bar-2, .bar-3, .bar-4, .bar-5 { border-radius: 10px; } .bar-5 { width: 70%; height: 18px; background-color: rgb(76, 175, 162); } .bar-4 { width: 50%; height: 18px; background-color: rgb(243, 222, 33); } .bar-3 { width: 20%; height: 18px; background-color: #12d400; } .bar-2 { width: 65%; height: 18px; background-color: #ff0055; } .bar-1 { width: 40%; height: 18px; background-color: #a836f4; } </style> </head> <body> <span class="rateHeader">User Rating</span> <span class="fa fa-star rated"></span> <span class="fa fa-star rated"></span> <span class="fa fa-star rated"></span> <span class="fa fa-star rated"></span> <span class="fa fa-star"></span> <p>3.9 average based on 200 foodies.</p> <div class="row"> <div class="data"> <div>5 star</div> </div> <div class="progressBar"> <div class="progressContainer"> <div class="bar-5"></div> </div> </div> <div class="data right"> <div>120</div> </div> <div class="data"> <div>4 star</div> </div> <div class="progressBar"> <div class="progressContainer"> <div class="bar-4"></div> </div> </div> <div class="data right"> <div>110</div> </div> <div class="data"> <div>3 star</div> </div> <div class="progressBar"> <div class="progressContainer"> <div class="bar-3"></div> </div> </div> <div class="data right"> <div>30</div> </div> <div class="data"> <div>2 star</div> </div> <div class="progressBar"> <div class="progressContainer"> <div class="bar-2"></div> </div> </div> <div class="data right"> <div>20</div> </div> <div class="data"> <div>1 star</div> </div> <div class="progressBar"> <div class="progressContainer"> <div class="bar-1"></div> </div> </div> <div class="data right"> <div>12</div> </div> </div> </body> </html>