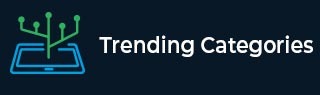
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to create a text input box with Pygame?
Pygame is a free and open-source library for developing multimedia applications like video games using Python. It includes graphics and sound libraries, which are very useful in designing video games. Pygame is built on top of the Simple DirectMedia Layer (SDL) library, which provides low-level access to hardware and input devices. Because Pygame is built on top of SDL, it provides a platform-independent interface for graphics, sound, and input handling. This means that you can write your game or multimedia application once and run it on multiple platforms, including Windows, Mac OS, and Linux.
To use pygame, one should have a basic understanding of Python language. By the end of this tutorial, we will be able to understand how Pygame works. It includes several functions to make video games and graphics. Before installing Pygame, Python should be installed in the system.
Example 1
In this example, we first imported Pygame and sys, then initialised all imported modules using ‘pygame.init()’ and defined ‘clock’ to refresh the frame in the given second. After then, we set the screen display mode and caption, following the font and text, and then we created a rectangle and set the color parameters. Next, we set the working flow of the input box by using several functions. Finally, we displayed it using the ‘pygame.display.flip()’ function.
import pygame,sys pygame.init() clock = pygame.time.Clock() screen= pygame.display.set_mode((500,500)) pygame.display.set_caption('text input') user_text= ' ' font = pygame.font.SysFont('frenchscript',32) input_rect = pygame.Rect(200,200,140,40) active=False color_ac=pygame.Color('green') color_pc=pygame.Color('red') color=color_pc active=False while True: for events in pygame.event.get(): if events.type==pygame.QUIT: pygame.quit() sys.exit() if events.type==pygame.MOUSEBUTTONDOWN: if input_rect.collidepoint(events.pos): active = True if events.type == pygame.KEYDOWN: if active == True: if events.key == pygame.K_BACKSPACE: user_text = user_text[:-1] else: user_text+=events.unicode screen.fill('blue') if active: color=color_ac else: color=color_pc pygame.draw.rect(screen,color,input_rect,2) text_surface = font.render(user_text,True,(255,255,255)) screen.blit(text_surface,(input_rect.x + 5, input_rect.y +5)) input_rect.w=max(100,text_surface.get_width() + 10) pygame.display.flip() clock.tick(60)
Output
Example 2
Here’s another similar example, where we created a text input box using the pygame module only, and then we defined other methods to create a text box. Finally, we displayed it using the ‘pygame.display()’ function.
import pygame pygame.init() screen = pygame.display.set_mode((700, 200)) clock = pygame.time.Clock() font = pygame.font.SysFont(None, 50) text = "" input_active = True run = True while run: clock.tick(60) for event in pygame.event.get(): if event.type == pygame.QUIT: run = False elif event.type == pygame.MOUSEBUTTONDOWN: input_active = True text = "" elif event.type == pygame.KEYDOWN and input_active: if event.key == pygame.K_RETURN: input_active = False elif event.key == pygame.K_BACKSPACE: text = text[:-1] else: text += event.unicode screen.fill('purple') text_surf = font.render(text, True, (255, 0, 0)) screen.blit(text_surf, text_surf.get_rect(center = screen.get_rect().center)) pygame.display.flip() pygame.quit() exit()
Output
Conclusion
We learned that Pygame is a popular library for creating video games and multimedia applications. Developers can create several games using this famous library. It provides an easy-to-use interface for creating and manipulating graphics. Anyone can use it to draw shapes, images, and animations on the screen. You can also use it to create visual effects such as particle systems and scrolling backgrounds. Overall, Pygame is a powerful and versatile library that can be used for various applications.
By learning Pygame, everyone can develop skills in graphics programming, sound programming, input handling, game development, and cross-platform development. With its easy-to-use interface and wide range of features, Pygame is a great choice for anyone interested in creating games or multimedia applications using Python.