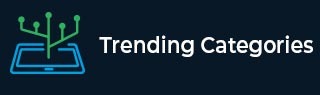
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to create a simple Recursive Function in Golang?
In this tutorial, we are going to see how we can write a simple recursive function Golang with the help of an algorithm and, examples. In the first example, we will print the number from 1 to N in ascending order similarly in the other example we are going to print the number from 1 to N in descending order.
In terms of programming, a function is a recursive function if we are calling the function in the same function and have a base condition. Whenever the base condition is satisfied the calling of the function is stopped and we start rolling back to the last step where the function is called.
Syntax
func functionName(arguments) returnType { // Base condition // Function calling according to the logic }
Example 1
In this example, we are going to see the recursive function to print the first 10 numbers in ascending order in Golang.
package main import ( // fmt package provides the function to print anything "fmt" ) func printAscendingOrder(number int) { // base condition of the function whenever the number becomes zero the function calls will stop if number == 0 { return } // calling the function within the function to make it recursive also, passing the number one lesser than the current value printAscendingOrder(number - 1) // printing the value of the number in // the current function calling the state fmt.Printf("%d ", number) } func main() { // declaring the variable var number int // initializing the variable number = 10 fmt.Println("Golang program to print the number in ascending order with the help of a simple recursive function.") // calling the recursive function printAscendingOrder(number) fmt.Println() }
Output
Golang program to print the number in ascending order with the help of a simple recursive function. 1 2 3 4 5 6 7 8 9 10
Example 2
In this example, we are going to see the recursive function to print the first 10 numbers in descending order in Golang.
package main import ( // fmt package provides the function to print anything "fmt" ) func printDescendingOrder(number int) { // base condition of the function whenever the number becomes zero the function calls will stop if number == 0 { return } // printing the value of the number in the current function calling the state fmt.Printf("%d ", number) // calling the function within the function to make it recursive also, passing the number one lesser than the current value printDescendingOrder(number - 1) } func main() { // declaring the variable var number int // initializing the variable number = 10 fmt.Println("Golang program to print the number in descending order with the help of a simple recursive function.") // calling the recursive function printDescendingOrder(number) fmt.Println() }
Output
Golang program to print the number in descending order with the help of a simple recursive function. 10 9 8 7 6 5 4 3 2 1
Conclusion
This is the way to create a simple recursive function in Golang with two examples where we have printed the numbers from 1 to N in ascending and descending order. To learn more about Golang you can explore these tutorials.