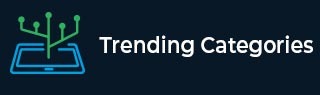
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to create a simple counter Using ReactJS?
Your online application can benefit greatly from adding interactivity by utilizing ReactJS to create a straightforward counter. In this tutorial, we will outline the procedures needed to build a straightforward counter using ReactJS and offer some sample code to get you started.
Step 1: Setting up the React Project
To get started, you will need to have a React project set up. If you don't already have one, you can use the create-react-app command to set up a new react project.
npx create-react-app counter-app cd counter-app npm start
This will set up a new project in a directory called counter-app, and it will also start a development server so we can see changes directly in the browser.
Step 2: Creating the Counter Component
Now that we have mustt project set up, we can create a new component for our counter. We will create a new file Counter.js in the src directory of our project.
Counter.js
import React, { useState } from 'react'; function Counter() { const [counter, setCounter] = useState(0); return ( <div className='counter-container'> <button onClick={() => setCounter(counter + 1)}>Increment</button> <button onClick={() => setCounter(counter - 1)}>Decrement</button> <button onClick={handleReset}>Reset</button> <p>Count: {counter}</p> </div> ); } export default Counter;
We are importing React and the useState hook from the react package into this code. We may add state to our component—in this case, the count—using the useState hook. When the Increment button is hit, we set the initial state to 0, update it with the updated count using the setCounter function, and then re-render the component. Similar to this, we decrement the count by one when we click the button.
Step 3: Adding the Counter to the App
Now that we have our counter component, we need to add it to our app. We will do this by importing the Counter component into the App.js file and adding it to the JSX.
App.js
import React from 'react'; import Counter from './Counter'; function App() { return ( <div> <Counter /> </div> ); } export default App;
Step 4: Styling the Counter
Finally, we can add some styling to our counter to make it look more visually appealing. We can do this by adding a CSS file and importing it into our Counter.js file.
import './Counter.css';
Create a Counter.css file and add the styles to it.
/* Counter.css */ button { padding: 10px 20px; background-color: #0052cc; color: white; border: none; cursor: pointer; margin: 10px; border-radius: 5px; font-size: 16px; transition: all 0.3s ease; }
This will give our counter some basic styling, such as a green background colour for the increment and decrement buttons and white text with a moving cursor to denote clickability. To make the buttons more dynamic, you can also add the states of hover, activity, and attention.
button:hover { background-color: #3e8e41; } button:active { transform: scale(0.95); } button:focus { outline: none; }
Step 5: Making the Counter Dynamic
To make the counter more dynamic, you can add an input field that allows the user to set the initial count. You can also add a reset button that allows the user to reset the count to the initial value.
Counter.js
import React, { useState } from 'react'; import './Counter.css'; function Counter() { const [counter, setCounter] = useState(0); const [initialCount, setInitialCount] = useState(0); const handleInitialCountChange = (event) => { setInitialCount(event.target.value); }; const handleReset = () => { setCounter(initialCount); }; return ( <div className='counter-container '> <button onClick={() => setCounter(counter + 1)}>Increment</button> <button onClick={() => setCounter(counter - 1)}>Decrement</button> <button onClick={handleReset}>Reset</button> <p >Count: {counter}</p> </div> ); } export default Counter;
This code now includes an input field where the user may enter the initial count and a reset button where they can return the count to its starting number. Additionally, we've included an event handler that modifies the initialCount state whenever the value of the input field changes.
Step 6: Making the Counter Responsive
Using CSS media queries, we can style the component differently depending on the screen size to make the counter responsive. On small displays, we can utilize flexbox to make the component occupy the entire width of its container.
Counter.css
button { padding: 10px 20px; background-color: #0052cc; color: white; border: none; cursor: pointer; margin: 10px; border-radius: 5px; font-size: 16px; transition: all 0.3s ease; } button:hover { background-color: #3e8e41; } button:active { transform: scale(0.95); } button:focus { outline: none; } .counter-container { display: flex; justify-content: center; flex-wrap: wrap; }
Output
With these steps, you should now have a fully functional and responsive counter in your ReactJS application. You can continue to customize and expand on this counter to fit the specific needs of your application.
You could also add the ability to save the count value in the local storage or in a database so that the count persists across page refreshes. You could also add the ability to set a maximum and minimum count limit and display an error message when the user tries to increment or decrement the count beyond the limit.
In addition, we can also add the ability to display a history of the count values and allow the user to go back and forth through the count history.
In this tutorial, we have developed a simple counter which can then be modified and improved to meet the particular requirements of the application. But bear in mind that there are other alternative ways to accomplish the same goal when building a counter in ReactJS; this is only one method.
Your online application can benefit greatly from adding interactivity by utilizing ReactJS to create a straightforward counter.