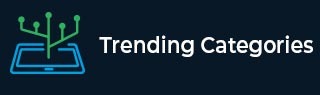
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to create a moving div using JavaScript?
A moving div is a web page element that can be caused to move across the screen, or change position on the screen, automatically. The position is changed by adjusting the left and top style properties. Creating a moving div using JavaScript is a relatively simple task. All that is required is a little bit of HTML, CSS, and JavaScript code. In this tutorial, we will go over how to create a moving div using JavaScript.
HTML Code
The first thing we need is some HTML code. We will create a div with an id of "movingDiv". Inside of this div, we will put some content. This content can be anything you want, but for this example, we will just put some text.
<div id="movingDiv"> This is my moving div! </div>
Now that we have our HTML code, we need some CSS code.
CSS Code
The CSS code is what will make our div actually move. We will set the position of our div to "relative". This will allow us to move our div around using JavaScript. We will also set the width and height of our div.
#movingDiv { position: relative; width: 200px; height: 200px; }
Now that we have our HTML and CSS code, we need some JavaScript code.
JavaScript Code
The JavaScript code is what will actually make our div move. We will use the setInterval function to move our div every 1000 milliseconds (1 second). We will also use the CSS properties "top" and "left" to move our div around.
var interval = setInterval(function() { var div = document.getElementById("movingDiv"); div.style.top = div.offsetTop + 1 + "px"; div.style.left = div.offsetLeft + 1 + "px"; }, 1000);
Example
Here is the full working code for this example −
<!doctype html> <html> <head> <style> #movingDiv { position: relative; width: 200px; height: 200px; } </style> </head> <body> <div id="movingDiv"> This is my moving div! </div> <script> var interval = setInterval(function() { var div = document.getElementById("movingDiv"); div.style.top = div.offsetTop + 1 + "px"; div.style.left = div.offsetLeft + 1 + "px"; }, 1000); </script> </body> </html>
A line-by-line explanation of the above code −
Line 1 − We start off by creating an HTML document.
Line 3 − We create a head element.
Line 4 − We create a style element. Inside this style element, we will put our CSS code.
Line 5 − We create a CSS rule for our div with an id of "movingDiv". We set the position to "relative". We also set the width and height of our div.
Line 12 − We create a body element. Inside of this body element, we will put our HTML code.
Line 13 − We create a div with an id of "movingDiv". Inside of this div, we put some text.
Line 14 − We create a script element. Inside this script element, we will put our JavaScript code.
Line 15 − We create a variable called "interval". We set this variable to the setInterval function. This function will move our div every 1000 milliseconds (1 second).
Line 16 − We create a variable called "div". We set this variable to the HTML element with an id of "movingDiv".
Line 17 − We use the CSS "top" property to move our div down 1 pixel.
Line 18 − We use the CSS "left" property to move our div to the right 1 pixel.
Line 22 − We end our HTML document.
In this tutorial, we went over how to create a moving div using JavaScript. We started off by creating some HTML code. We then created some CSS code. Finally, we created some JavaScript code.