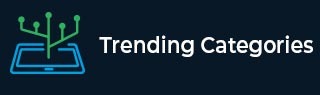
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to create a list in Kotlin?
A list is a collection to hold same type of data in a single variable. Kotlin does not provide any dedicated literal to create a collection. As per the documentation of Kotlin, a List is an ordered collection with access to elements by indices.
In Kotlin, we do have two different kinds of collections; one is read-only which is known as immutable collection and the other type of collection is where we can have a write facility as well which is known as mutable collection.
In this article, we will see how we can create these two types of lists and manipulate the same using Kotlin library function.
Example - Immutable list in Kotlin
In the following example, we will create an immutable list and we will try to manipulate its values.
fun main(args: Array<String>) { val myList = listOf("apple","mango","bread","Milk") println(myList) // myList.add("butter") }
Output
On execution, it will generate the following output −
[apple, mango, bread, Milk]
Note that we cannot add any value to an immutable list. Suppose you try to insert a new value in the list, like −
myList.add("butter")
Then, it will produce an error because we cannot manipulate an immutable list.
Example - Mutable list in Kotlin
In this example, we will create a mutable list and we will try to manipulate its values. For this example, we have used mutableListOf() but we can also use arrayListOf(), which can also create an mutable list.
fun main(args: Array<String>) { val myList = mutableListOf("apple","mango","bread","Milk") println("The original list:" + myList) myList.add("butter") println("The modified list:" + myList) }
Output
It will produce the following output −
The original list:[apple, mango, bread, Milk] The modified list:[apple, mango, bread, Milk, butter]
Observe that we were being able to modify the original list because it is possible to manipulate the values of a mutable list.