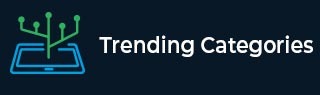
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to create a JavaScript callback for knowing if an image is loaded?
Strong programming languages like JavaScript are frequently used to build interactive, dynamic web pages. The loading of images on a website is one of JavaScript's most popular uses. However, loading images might be challenging, particularly when determining whether a load is complete. In this article, we'll go through how to make a JavaScript callback that detects if an image has loaded or not.
What is a callback function?
A callback function is a function that is invoked after the initial function has finished running and is supplied as an argument to another function. To put it another way, a callback function is one that is carried out after another function has completed its execution. JavaScript's powerful capability that enables the creation of more intricate and dynamic web pages.
Why use a callback for image loading?
The moment the element is discovered in the HTML code; the browser begins loading the image when you upload an image to a website. The image might not have finished loading by the time the browser reaches the end of the HTML code. If you attempt to access the image before it has finished loading, this could lead to issues. For instance, if you attempt to retrieve an image's width and height before it has finished loading, you may receive inaccurate results.
Use a callback method that is executed after the image has finished loading to get around this issue. By doing this, you can make sure that the image has completely loaded before attempting to access its properties.
How to create a callback for image loading?
There are several ways to create a callback for image loading
Using onload event of the <img> tag
Using the onload event of the <img> tag is the most popular approach. When an image has completely loaded, the onload event is started. You can attach a callback function to this event by setting the onload attribute of the <img> tag to the name of the callback function.
As an illustration, suppose you want to develop a callback function that displays the width and height of the image and you have an <img> element with the id "myImage".
Example
The following code can be used to accomplish this −
<html> <body> <div id="width-display"></div> <div id="height-display"></div> <div id= "info"></div> <img id="myImage" onload="imageLoaded()" src="https://picsum.photos/600/400" /> <div id="data-display"></div> <div id="error-display"></div> <script> function imageLoaded() { var img = document.getElementById("myImage"); var widthDisplay = document.getElementById("width-display"); widthDisplay.innerHTML = "Width: " + img.width; var heightDisplay = document.getElementById("height-display"); heightDisplay.innerHTML = "Height: " + img.height; document.getElementById("info").innerHTML = "Image is loaded successfully."; } </script> </body> </html>
Image will be different every time you refresh the page.
In this example, the function imageLoaded() is attached to the onload event of the tag. When the image is fully loaded, the function is executed and it displays the width and height of the image.
Using the addEventListener method
The addEventListener method can also be used to construct a callback for image loading. Using this method, you can specify the callback function to be used when an event is triggered and attach an event listener to an element. This technique can be used to add an event listener to the "load" event of the <img> tag.
Example
Using the addEventListener method, you could write the following code to create a callback for image loading −
<html> <body> <img id="myImage" src="https://picsum.photos/200/300" /> <p id="width-display"></p> <p id="height-display"></p> <div id= "info"></div> <script type="text/javascript"> var img = document.getElementById("myImage"); img.addEventListener("load", function(){ var widthDisplay = document.getElementById("width-display"); widthDisplay.innerHTML = "Width: " + img.width; var heightDisplay = document.getElementById("height-display"); heightDisplay.innerHTML = "Height: " + img.height; document.getElementById("info").innerHTML = "Image is loaded successfully."; }); </script> </body> </html>
In this example, the addEventListener method is used to attach the imageLoaded function to the "load" event of the <img> tag. When the image is fully loaded, the function is executed and it displays the width and height of the image.
Using the setTimeout function
The two methods most frequently used to generate a callback for image loading are the onload event and the addEventListener function. However, There are various approaches to doing this.
Example
For example, you can check if the image has loaded using the setTimeout method after a predetermined period of time.
<html> <head> <script> function checkImageLoaded() { var img = document.getElementById("myImage"); if (img.complete) { document.getElementById("width").innerHTML = "Width: " + img.width; document.getElementById("height").innerHTML = "Height: " + img.height; document.getElementById("info").innerHTML = "Image is loaded successfully."; } else { setTimeout(checkImageLoaded, 50); } } </script> </head> <body> <img id="myImage" onload="checkImageLoaded()" src="https://picsum.photos/100/100" /> <p id="width"></p> <p id="height"></p> <p id="info"></p> </body> </html>
This example repeatedly calls the checkImageLoaded method every 50 milliseconds until the image has loaded completely.
In this tutorial, we've covered the value of using callback functions for loading images as well as several callback function creation techniques. You can quickly develop a callback function for picture loading by following these instructions, which will also guarantee that your photos are fully loaded before being accessible.