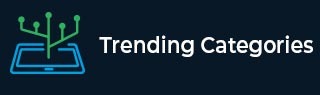
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to Create a Hotkey in Python?
Hotkeys are a convenient way to automate repetitive tasks in Python programs. Hotkeys allow users to perform an action quickly and easily, without having to navigate through menus or use a mouse. In this tutorial, we will discuss how to create a hotkey in Python using the keyboard library.
This keyboard library provides a simple and easy-to-use API for registering hotkeys and responding to keyboard events. By creating hotkeys in your Python programs, you can enhance the user experience and improve productivity by allowing users to perform tasks quickly and efficiently.
We will cover the approach and two code examples with comments and explanations to help you understand the process better.
Approach to Create a Hotkey in Python
To create a hotkey in Python, we will be using the keyboard library. This library allows us to listen for keyboard events and perform actions when specific keys are pressed. The keyboard library is a cross-platform library, which means that it works on both Windows and Linux.
The general approach for creating a hotkey in Python using the keyboard library is −
Import the keyboard library
Define a function that will be executed when the hotkey is pressed
Register the hotkey using the keyboard library
Listen for keyboard events
Now, let's move on to the code examples.
Example 1
In this code example, we will create a hotkey that will print a message to the console when the "space" key is pressed.
Consider the code shown below.
# import the keyboard module import keyboard # define a function that will be executed when the hotkey is pressed def hotkey_pressed(): print("Space was pressed!") # register the hotkey using the keyboard library keyboard.add_hotkey('space', hotkey_pressed) # wait for keyboard events keyboard.wait()
Explanation
Import the keyboard library. The hotkey_pressed() function is defined to print a message to the console when the hotkey is pressed.
The keyboard.add_hotkey() function is used to register the hotkey. This function takes two arguments: the hotkey combination (in this case, the "space" key) and the function that will be executed when the hotkey is pressed (in this case, hotkey_pressed() function).
Finally, the keyboard.wait() function is called to keep the program running and listening for keyboard events.
To run the above code, we first need to install the keyboard library in our machine, and for that, we can use the command shown below
pip3 install keyboard
Output
Now, execute the code and then press the space button on the keyboard. You will get the following output as response −
Space was pressed!
Example 2
In this code example, we will create a hotkey that will print a message to the console when the " " key is pressed.
Consider the code shown below.
# import the keyboard module import keyboard # define a function that will be executed when the hotkey is pressed def hotkey_pressed(): print("Space was pressed!") # register the hotkey using the keyboard library keyboard.add_hotkey(' ', hotkey_pressed) # wait for keyboard events keyboard.wait()
Explanation
Import the keyboard module. Define a function named hotkey_pressed() that will be executed when the hotkey is pressed. In this case, the function prints the message "Space was pressed!" to the console.
Register the hotkey using the keyboard.add_hotkey() function. The hotkey combination is the space bar and the function to be executed when the hotkey is pressed is hotkey_pressed().
Wait for keyboard events using the keyboard.wait() function, which keeps the program running and listening for keyboard events.
When the spacebar is pressed, the function hotkey_pressed() is executed and the message "Space was pressed!" is printed to the console. The program continues to wait for keyboard events until it is manually stopped.
Output
Now, execute the code and then press the space button on the keyboard. You will get the following output as response:
Space was pressed!
Conclusion
Creating a hotkey in Python using the keyboard library is a powerful way to automate repetitive tasks.
We discussed the general approach to creating a hotkey, and then provided two code examples with explanations. With these examples, you should have a good understanding of how to create hotkeys in Python and how to use the keyboard library.