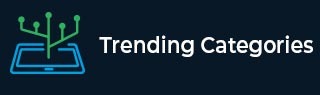
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to create a ChoiceDialog in JavaFX?
A ChoiceDialog is a dialog box that shows a list of choices from which you can select one. You can create a choice dialog by instantiating the javafx.scene.control.ChoiceDialog class.
Example
The following Example demonstrates the creation of a ChoiceDialog.
import javafx.application.Application; import javafx.collections.ObservableList; import javafx.geometry.Insets; import javafx.scene.Scene; import javafx.scene.control.Button; import javafx.scene.control.ChoiceDialog; import javafx.scene.layout.HBox; import javafx.scene.text.Font; import javafx.scene.text.FontPosture; import javafx.scene.text.FontWeight; import javafx.scene.text.Text; import javafx.stage.Stage; public class ChoiceDialogExample extends Application { public void start(Stage stage) { //Creating a choice box ChoiceDialog<String> choiceDialog = new ChoiceDialog<String>("English"); //Retrieving the observable list ObservableList<String> list = choiceDialog.getItems(); //Adding items to the list list.add("English"); list.add("Hindi"); list.add("Telugu"); list.add("Tamil"); //Setting the display text Text txt = new Text("Click the button to show the choice dialog"); Font font = Font.font("verdana", FontWeight.BOLD, FontPosture.REGULAR, 12); txt.setFont(font); //Creating a button Button button = new Button("Show Dialog"); //Showing the choice dialog on clicking the button button.setOnAction(e -> { choiceDialog.showAndWait(); }); //Adding the choice box to the group HBox layout = new HBox(25); layout.getChildren().addAll(txt, button); layout.setPadding(new Insets(15, 50, 50, 50)); layout.setStyle("-fx-background-color: BEIGE"); //Setting the stage Scene scene = new Scene(layout, 595, 300); stage.setTitle("Choice Dialog"); stage.setScene(scene); stage.show(); } public static void main(String args[]){ launch(args); } }
Output
Advertisements